Building a Telegram Bot with FastAPI and Vue.js: A Step-by-Step Guide
January 13, 2025, 4:36 pm
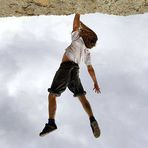
Location: United States, California, San Francisco
Employees: 51-200
Founded date: 2015
Total raised: $50M
In the digital age, convenience is king. Imagine a world where booking a doctor's appointment is as easy as sending a message. This is the promise of a Telegram bot integrated with a MiniApp, powered by FastAPI and Vue.js. This article will guide you through the creation of a robust backend and a sleek frontend for a fictional clinic called "Health Plus."
### The Vision
The goal is simple: create a Telegram bot that allows users to book appointments with doctors at their convenience. The bot will serve as a bridge between patients and healthcare providers, making the process seamless and efficient.
### The Tech Stack
We will use two main frameworks: FastAPI for the backend and Vue.js for the frontend. FastAPI is a powerful Python framework that excels in building APIs quickly and efficiently. Vue.js, on the other hand, is a progressive JavaScript framework that makes building user interfaces a breeze.
Additionally, we will incorporate several libraries to enhance our project:
- **FastAPI**: The backbone of our API.
- **SQLAlchemy**: For database interactions.
- **Apscheduler**: To manage scheduled tasks, like sending appointment reminders.
- **HTTPX**: For communicating with the Telegram API.
- **Vue.js**: To create a dynamic frontend.
- **Tailwind CSS**: For styling without the hassle of writing CSS from scratch.
### Project Structure
Our project will consist of three main components:
1. **API**: The heart of our application, handling data interactions and business logic.
2. **Telegram Bot**: A standalone implementation that interacts with users.
3. **Frontend**: The user interface for booking appointments.
### Development Steps
#### Step 1: Setting Up the Environment
First, we need to create a new project in our favorite IDE. I recommend using PyCharm for Python development. After setting up the project, we will create a virtual environment and install the necessary libraries using a `requirements.txt` file.
```plaintext
fastapi==0.115.0
pydantic==2.9.2
uvicorn==0.31.0
sqlalchemy==2.0.35
httpx==0.28.1
apscheduler==3.11.0
```
Run the command:
```bash
pip install -r requirements.txt
```
#### Step 2: Configuring Environment Variables
Next, we need to set up our environment variables. Create a `.env` file in the root of the project. This file will store sensitive information like the bot token and database URL.
```plaintext
BOT_TOKEN=your_bot_token
DATABASE_URL=sqlite:///data/db.sqlite3
```
#### Step 3: Structuring the Project
Create a folder structure that separates concerns. Here’s a suggested layout:
```
app/
├── api/
├── dao/
├── tg_bot/
├── static/
└── main.py
```
- **api/**: Contains the API endpoints.
- **dao/**: Handles database interactions.
- **tg_bot/**: Contains the logic for the Telegram bot.
- **static/**: For static files like images.
#### Step 4: Database Setup
Using SQLAlchemy, we will define our database models. Create a `models.py` file in the `dao` directory. Here, we will define models for users, doctors, and appointments.
```python
from sqlalchemy import Column, Integer, String, ForeignKey
from sqlalchemy.orm import relationship
from app.dao.database import Base
class User(Base):
__tablename__ = "users"
id = Column(Integer, primary_key=True)
telegram_id = Column(Integer, unique=True)
username = Column(String)
class Doctor(Base):
__tablename__ = "doctors"
id = Column(Integer, primary_key=True)
name = Column(String)
specialization = Column(String)
class Booking(Base):
__tablename__ = "bookings"
id = Column(Integer, primary_key=True)
user_id = Column(Integer, ForeignKey("users.id"))
doctor_id = Column(Integer, ForeignKey("doctors.id"))
```
#### Step 5: Building the API
Now, let’s create the API endpoints. In the `api` directory, create a file called `endpoints.py`. Here, we will define routes for booking appointments, viewing doctors, and managing user data.
```python
from fastapi import APIRouter
from app.dao.models import User, Doctor, Booking
router = APIRouter()
@router.post("/book")
async def book_appointment(user_id: int, doctor_id: int):
# Logic to book an appointment
pass
@router.get("/doctors")
async def get_doctors():
# Logic to retrieve doctors
pass
```
#### Step 6: Implementing the Telegram Bot
In the `tg_bot` directory, create a file called `bot.py`. This file will handle incoming messages and interact with the API.
```python
import httpx
from fastapi import FastAPI
app = FastAPI()
@app.post("/webhook")
async def handle_webhook(update: dict):
# Logic to handle incoming Telegram messages
pass
```
#### Step 7: Frontend Development
Now, let’s shift our focus to the frontend. Using Vue.js, we will create a user-friendly interface. Start by setting up a new Vue project.
```bash
vue create frontend
```
In the Vue project, create components for selecting a doctor, booking an appointment, and viewing existing bookings. Use Axios to communicate with the FastAPI backend.
#### Step 8: Deployment
Finally, we need to deploy our application. For simplicity, we can use a cloud service like Heroku or DigitalOcean. The deployment process typically involves:
1. Pushing the backend code to the cloud.
2. Configuring environment variables on the cloud platform.
3. Deploying the frontend code.
### Conclusion
Building a Telegram bot with FastAPI and Vue.js is a rewarding endeavor. It combines the power of modern web technologies with the convenience of instant messaging. By following this guide, you can create a functional bot that simplifies the appointment booking process.
In a world where time is precious, this bot is a small step towards making healthcare more accessible. So, roll up your sleeves and start coding! The future of healthcare is just a message away.
### The Vision
The goal is simple: create a Telegram bot that allows users to book appointments with doctors at their convenience. The bot will serve as a bridge between patients and healthcare providers, making the process seamless and efficient.
### The Tech Stack
We will use two main frameworks: FastAPI for the backend and Vue.js for the frontend. FastAPI is a powerful Python framework that excels in building APIs quickly and efficiently. Vue.js, on the other hand, is a progressive JavaScript framework that makes building user interfaces a breeze.
Additionally, we will incorporate several libraries to enhance our project:
- **FastAPI**: The backbone of our API.
- **SQLAlchemy**: For database interactions.
- **Apscheduler**: To manage scheduled tasks, like sending appointment reminders.
- **HTTPX**: For communicating with the Telegram API.
- **Vue.js**: To create a dynamic frontend.
- **Tailwind CSS**: For styling without the hassle of writing CSS from scratch.
### Project Structure
Our project will consist of three main components:
1. **API**: The heart of our application, handling data interactions and business logic.
2. **Telegram Bot**: A standalone implementation that interacts with users.
3. **Frontend**: The user interface for booking appointments.
### Development Steps
#### Step 1: Setting Up the Environment
First, we need to create a new project in our favorite IDE. I recommend using PyCharm for Python development. After setting up the project, we will create a virtual environment and install the necessary libraries using a `requirements.txt` file.
```plaintext
fastapi==0.115.0
pydantic==2.9.2
uvicorn==0.31.0
sqlalchemy==2.0.35
httpx==0.28.1
apscheduler==3.11.0
```
Run the command:
```bash
pip install -r requirements.txt
```
#### Step 2: Configuring Environment Variables
Next, we need to set up our environment variables. Create a `.env` file in the root of the project. This file will store sensitive information like the bot token and database URL.
```plaintext
BOT_TOKEN=your_bot_token
DATABASE_URL=sqlite:///data/db.sqlite3
```
#### Step 3: Structuring the Project
Create a folder structure that separates concerns. Here’s a suggested layout:
```
app/
├── api/
├── dao/
├── tg_bot/
├── static/
└── main.py
```
- **api/**: Contains the API endpoints.
- **dao/**: Handles database interactions.
- **tg_bot/**: Contains the logic for the Telegram bot.
- **static/**: For static files like images.
#### Step 4: Database Setup
Using SQLAlchemy, we will define our database models. Create a `models.py` file in the `dao` directory. Here, we will define models for users, doctors, and appointments.
```python
from sqlalchemy import Column, Integer, String, ForeignKey
from sqlalchemy.orm import relationship
from app.dao.database import Base
class User(Base):
__tablename__ = "users"
id = Column(Integer, primary_key=True)
telegram_id = Column(Integer, unique=True)
username = Column(String)
class Doctor(Base):
__tablename__ = "doctors"
id = Column(Integer, primary_key=True)
name = Column(String)
specialization = Column(String)
class Booking(Base):
__tablename__ = "bookings"
id = Column(Integer, primary_key=True)
user_id = Column(Integer, ForeignKey("users.id"))
doctor_id = Column(Integer, ForeignKey("doctors.id"))
```
#### Step 5: Building the API
Now, let’s create the API endpoints. In the `api` directory, create a file called `endpoints.py`. Here, we will define routes for booking appointments, viewing doctors, and managing user data.
```python
from fastapi import APIRouter
from app.dao.models import User, Doctor, Booking
router = APIRouter()
@router.post("/book")
async def book_appointment(user_id: int, doctor_id: int):
# Logic to book an appointment
pass
@router.get("/doctors")
async def get_doctors():
# Logic to retrieve doctors
pass
```
#### Step 6: Implementing the Telegram Bot
In the `tg_bot` directory, create a file called `bot.py`. This file will handle incoming messages and interact with the API.
```python
import httpx
from fastapi import FastAPI
app = FastAPI()
@app.post("/webhook")
async def handle_webhook(update: dict):
# Logic to handle incoming Telegram messages
pass
```
#### Step 7: Frontend Development
Now, let’s shift our focus to the frontend. Using Vue.js, we will create a user-friendly interface. Start by setting up a new Vue project.
```bash
vue create frontend
```
In the Vue project, create components for selecting a doctor, booking an appointment, and viewing existing bookings. Use Axios to communicate with the FastAPI backend.
#### Step 8: Deployment
Finally, we need to deploy our application. For simplicity, we can use a cloud service like Heroku or DigitalOcean. The deployment process typically involves:
1. Pushing the backend code to the cloud.
2. Configuring environment variables on the cloud platform.
3. Deploying the frontend code.
### Conclusion
Building a Telegram bot with FastAPI and Vue.js is a rewarding endeavor. It combines the power of modern web technologies with the convenience of instant messaging. By following this guide, you can create a functional bot that simplifies the appointment booking process.
In a world where time is precious, this bot is a small step towards making healthcare more accessible. So, roll up your sleeves and start coding! The future of healthcare is just a message away.