The RAM Myth: Unpacking the Illusions of Memory Performance
December 24, 2024, 4:23 am
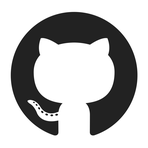
Location: United States, California, San Francisco
Employees: 1001-5000
Founded date: 2008
Total raised: $350M
In the world of computing, myths often cloud the truth. One such myth is the belief that modern RAM operates like an ideal memory system. This notion leads many to think that simply having more RAM guarantees better performance. However, the reality is far more complex.
RAM, or Random Access Memory, is not a magic wand. It’s a tool, and like any tool, its effectiveness depends on how it’s used. The idea that cache memory is a simple optimization for small data sets is misleading. If data fits into the L2 cache, it processes quickly. If not, performance can plummet.
Consider this: when we access data, we’re not just pulling it from a shelf. We’re navigating a labyrinth. The faster we can find our way, the better our performance. But when the data is scattered, even the best algorithms can struggle.
Take a look at a simple algorithm. It’s designed to group elements based on their attributes. In pseudocode, it looks like this:
```python
groups = [[] for _ in range(n_groups)]
for element in elements:
groups[element.group].append(element)
```
This approach is linear, meaning it scales well. But when the number of groups is high, performance can take a hit. Some slower algorithms can actually segment data more efficiently. This is especially true in disk databases, but surprisingly, it applies to RAM as well.
The problem arises with cache misses. Each time we access memory randomly, we risk a miss. To mitigate this, we can sort our data before processing. By ordering elements by group, we can significantly reduce cache misses.
Sorting takes time, but it pays off. Once sorted, we can group elements efficiently:
```python
elements.sort(key=lambda element: element.group)
groups = [group_elements for _, group_elements in itertools.groupby(elements, key=lambda element: element.group)]
```
This technique is not just a neat trick; it’s a necessity for large data sets. When data exceeds cache size, performance gains become crucial.
Sorting algorithms that consider cache efficiency exist. Among them, radix sort stands out. It’s particularly effective for integer data. In Rust, the `radsort` implementation shines.
As data volumes grow, the optimized code outperforms naive algorithms. But there’s more to the story. Generators can streamline the process. They allow us to yield groups without storing everything in memory at once.
Consider this generator for radix sort:
```python
def radix_sort(elements, bits=32):
if len(elements) <= 1 or bits <= 0:
yield from elements
return
buckets = [[] for _ in range(256)]
for element in elements:
buckets[(element.index >> max(0, bits - 8)) & 0xff].append(element)
for bucket in buckets:
yield from radix_sort(bucket, bits - 8)
```
This approach minimizes memory overhead. We can even eliminate the need for a separate grouping step.
Yet, there’s a catch. The code frequently reallocates memory for buckets, which can be detrimental to cache performance. To combat this, we can pre-calculate sizes:
```python
sizes = Counter(map(get_bucket, elements))
buckets = [reserve(sizes[i]) for i in range(256)]
```
This requires two passes, but it’s worth it. If we estimate bucket sizes based on input data, we can reserve space more effectively.
Now, let’s introduce a `Bucket` class to manage our memory:
```python
class Bucket:
def __init__(self, capacity):
self.reserved = reserve(capacity)
self.leftovers = []
def append(self, element):
if len(self.reserved) < self.reserved.capacity():
self.reserved.append(element)
else:
self.leftovers.append(element)
def __len__(self):
return len(self.reserved) + len(self.leftovers)
def __iter__(self):
yield from self.reserved
yield from self.leftovers
```
This structure allows us to handle overflow efficiently. The majority of elements will fit into reserved space, minimizing reallocations.
Now, let’s talk about optimization. When dealing with small data sets, switching to a straightforward algorithm can boost performance. This is especially true for radix sort, which can be recursive.
At each recursion level, we can check if the data size is small enough to warrant a simpler approach:
```python
if len(elements) <= CUTOFF or bits <= 8:
counts = [0] * 256
for element in elements:
counts[element.index & 0xff] += 1
groups = [[] for _ in range(256)]
for element in elements:
groups[get_bucket(element)].append(element)
for group in groups:
if group:
yield group
```
Finding the optimal cutoff value is crucial. It varies by machine and depends on cache speeds and data types. Benchmarking in the runtime environment is the best way to determine this.
Finally, let’s discuss benchmarks. We’ll analyze a set of random 64-bit integers, grouping them by a simple hash. We’ll compare a naive algorithm with our optimized radix sort.
The results are telling. The optimized algorithm outperforms the naive one as data volume increases. Both approaches eventually plateau, but the gains can be significant—between 2.5 to 9 times, depending on the machine.
So, is it worth the effort? If performance is critical, especially with large data sets, these optimizations are invaluable. But remember, with every optimization comes complexity.
In the end, the lesson is clear. Just as we wouldn’t treat disk data like RAM, we shouldn’t assume more RAM equals better performance. When dealing with large data sets, consider segmentation and external memory algorithms. The right approach can make all the difference.
RAM, or Random Access Memory, is not a magic wand. It’s a tool, and like any tool, its effectiveness depends on how it’s used. The idea that cache memory is a simple optimization for small data sets is misleading. If data fits into the L2 cache, it processes quickly. If not, performance can plummet.
Consider this: when we access data, we’re not just pulling it from a shelf. We’re navigating a labyrinth. The faster we can find our way, the better our performance. But when the data is scattered, even the best algorithms can struggle.
Take a look at a simple algorithm. It’s designed to group elements based on their attributes. In pseudocode, it looks like this:
```python
groups = [[] for _ in range(n_groups)]
for element in elements:
groups[element.group].append(element)
```
This approach is linear, meaning it scales well. But when the number of groups is high, performance can take a hit. Some slower algorithms can actually segment data more efficiently. This is especially true in disk databases, but surprisingly, it applies to RAM as well.
The problem arises with cache misses. Each time we access memory randomly, we risk a miss. To mitigate this, we can sort our data before processing. By ordering elements by group, we can significantly reduce cache misses.
Sorting takes time, but it pays off. Once sorted, we can group elements efficiently:
```python
elements.sort(key=lambda element: element.group)
groups = [group_elements for _, group_elements in itertools.groupby(elements, key=lambda element: element.group)]
```
This technique is not just a neat trick; it’s a necessity for large data sets. When data exceeds cache size, performance gains become crucial.
Sorting algorithms that consider cache efficiency exist. Among them, radix sort stands out. It’s particularly effective for integer data. In Rust, the `radsort` implementation shines.
As data volumes grow, the optimized code outperforms naive algorithms. But there’s more to the story. Generators can streamline the process. They allow us to yield groups without storing everything in memory at once.
Consider this generator for radix sort:
```python
def radix_sort(elements, bits=32):
if len(elements) <= 1 or bits <= 0:
yield from elements
return
buckets = [[] for _ in range(256)]
for element in elements:
buckets[(element.index >> max(0, bits - 8)) & 0xff].append(element)
for bucket in buckets:
yield from radix_sort(bucket, bits - 8)
```
This approach minimizes memory overhead. We can even eliminate the need for a separate grouping step.
Yet, there’s a catch. The code frequently reallocates memory for buckets, which can be detrimental to cache performance. To combat this, we can pre-calculate sizes:
```python
sizes = Counter(map(get_bucket, elements))
buckets = [reserve(sizes[i]) for i in range(256)]
```
This requires two passes, but it’s worth it. If we estimate bucket sizes based on input data, we can reserve space more effectively.
Now, let’s introduce a `Bucket` class to manage our memory:
```python
class Bucket:
def __init__(self, capacity):
self.reserved = reserve(capacity)
self.leftovers = []
def append(self, element):
if len(self.reserved) < self.reserved.capacity():
self.reserved.append(element)
else:
self.leftovers.append(element)
def __len__(self):
return len(self.reserved) + len(self.leftovers)
def __iter__(self):
yield from self.reserved
yield from self.leftovers
```
This structure allows us to handle overflow efficiently. The majority of elements will fit into reserved space, minimizing reallocations.
Now, let’s talk about optimization. When dealing with small data sets, switching to a straightforward algorithm can boost performance. This is especially true for radix sort, which can be recursive.
At each recursion level, we can check if the data size is small enough to warrant a simpler approach:
```python
if len(elements) <= CUTOFF or bits <= 8:
counts = [0] * 256
for element in elements:
counts[element.index & 0xff] += 1
groups = [[] for _ in range(256)]
for element in elements:
groups[get_bucket(element)].append(element)
for group in groups:
if group:
yield group
```
Finding the optimal cutoff value is crucial. It varies by machine and depends on cache speeds and data types. Benchmarking in the runtime environment is the best way to determine this.
Finally, let’s discuss benchmarks. We’ll analyze a set of random 64-bit integers, grouping them by a simple hash. We’ll compare a naive algorithm with our optimized radix sort.
The results are telling. The optimized algorithm outperforms the naive one as data volume increases. Both approaches eventually plateau, but the gains can be significant—between 2.5 to 9 times, depending on the machine.
So, is it worth the effort? If performance is critical, especially with large data sets, these optimizations are invaluable. But remember, with every optimization comes complexity.
In the end, the lesson is clear. Just as we wouldn’t treat disk data like RAM, we shouldn’t assume more RAM equals better performance. When dealing with large data sets, consider segmentation and external memory algorithms. The right approach can make all the difference.