Unlocking the Power of Pre-trained Neural Networks in Java Projects
December 19, 2024, 3:45 pm
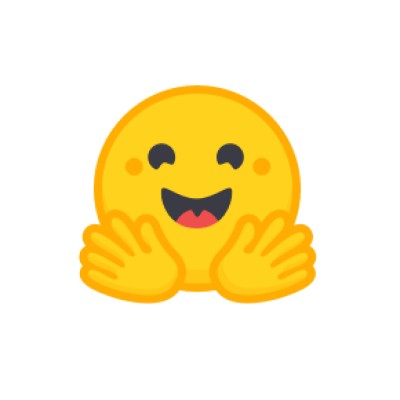
Location: Australia, New South Wales, Concord
Employees: 51-200
Founded date: 2016
Total raised: $494M
In the fast-paced world of technology, time is money. Developers are always on the lookout for ways to speed up their projects without sacrificing quality. Enter pre-trained neural networks. These models are like seasoned chefs who have perfected their recipes. Instead of starting from scratch, developers can leverage these models to whip up solutions quickly and efficiently.
Pre-trained models, especially in the realm of Natural Language Processing (NLP), have revolutionized how we handle text data. One shining example is Named Entity Recognition (NER). This task involves identifying and classifying key elements in text, such as names, locations, and organizations. Imagine a detective sifting through a pile of documents, searching for clues. NER does just that, but at lightning speed.
Consider a simple sentence: "John Doe works at TechCorp and lives in New York." A well-trained NER model can instantly tag "John Doe" as a person, "TechCorp" as an organization, and "New York" as a location. This capability is invaluable for businesses looking to analyze customer feedback, extract insights, or automate responses.
The beauty of using pre-trained models lies in their accessibility. Libraries like SpaCy, Stanford CoreNLP, and Hugging Face Transformers offer robust solutions that can be integrated into various programming environments, including Java. Each of these libraries has its strengths. SpaCy is known for its speed and multi-language support, while Stanford CoreNLP excels in handling complex linguistic structures. Hugging Face Transformers brings high accuracy with models like BERT and GPT, making it a favorite among developers.
For Java developers, integrating these models can seem daunting. However, tools like DeepPavlov and ONNX Runtime simplify the process. DeepPavlov specializes in Russian language tasks, making it a go-to for projects targeting that demographic. ONNX Runtime, on the other hand, allows for efficient execution of models across different platforms, ensuring that performance is never compromised.
To illustrate the integration process, let’s consider a practical example. Imagine you have a database filled with articles, and you want to extract information about mentioned people, places, and organizations. By employing a pre-trained NER model, you can automate this task, saving countless hours of manual labor. The steps are straightforward:
1.Set Up Your Environment
Pre-trained models, especially in the realm of Natural Language Processing (NLP), have revolutionized how we handle text data. One shining example is Named Entity Recognition (NER). This task involves identifying and classifying key elements in text, such as names, locations, and organizations. Imagine a detective sifting through a pile of documents, searching for clues. NER does just that, but at lightning speed.
Consider a simple sentence: "John Doe works at TechCorp and lives in New York." A well-trained NER model can instantly tag "John Doe" as a person, "TechCorp" as an organization, and "New York" as a location. This capability is invaluable for businesses looking to analyze customer feedback, extract insights, or automate responses.
The beauty of using pre-trained models lies in their accessibility. Libraries like SpaCy, Stanford CoreNLP, and Hugging Face Transformers offer robust solutions that can be integrated into various programming environments, including Java. Each of these libraries has its strengths. SpaCy is known for its speed and multi-language support, while Stanford CoreNLP excels in handling complex linguistic structures. Hugging Face Transformers brings high accuracy with models like BERT and GPT, making it a favorite among developers.
For Java developers, integrating these models can seem daunting. However, tools like DeepPavlov and ONNX Runtime simplify the process. DeepPavlov specializes in Russian language tasks, making it a go-to for projects targeting that demographic. ONNX Runtime, on the other hand, allows for efficient execution of models across different platforms, ensuring that performance is never compromised.
To illustrate the integration process, let’s consider a practical example. Imagine you have a database filled with articles, and you want to extract information about mentioned people, places, and organizations. By employing a pre-trained NER model, you can automate this task, saving countless hours of manual labor. The steps are straightforward:
1.
Set Up Your Environment: Start by adding the necessary dependencies to your Java project. For instance, include ONNX Runtime in your `pom.xml` file.
2. Download the Model: Obtain a pre-trained NER model, such as the one from DeepPavlov.
3. Write the Code: Implement the logic to load the model, tokenize your input text, and run the model to get predictions.
Here’s a snippet to get you started:
```java
import ai.onnxruntime.*;
import java.util.*;
public class NERExample {
public static void main(String[] args) throws OrtException {
String modelPath = "models/deeppavlov_ner_onnx_model.onnx";
OrtEnvironment env = OrtEnvironment.getEnvironment();
OrtSession session = env.createSession(modelPath, new OrtSession.SessionOptions());
String text = "John Doe works at TechCorp and lives in New York.";
String[] tokens = tokenize(text);
long[][] inputIds = prepareInputIds(tokens);
long[][] attentionMask = prepareAttentionMask(tokens);
OrtSession.Result result = session.run(Map.of("input_ids", createTensor(env, inputIds), "attention_mask", createTensor(env, attentionMask)));
processResults(result);
}
// Additional methods for tokenization, input preparation, and result processing
}
```
This code snippet is a mere starting point. It showcases how to load a model and prepare input data. The real magic happens in the details—tokenization, attention masks, and interpreting the model's output.
The advantages of using pre-trained models are clear. They save time, enhance accuracy, and allow developers to focus on higher-level tasks rather than getting bogged down in the intricacies of model training. With the right tools, integrating these models into Java projects becomes a seamless experience.
However, challenges remain. Developers must understand the underlying data structures, such as tensors, which are essential for processing inputs and outputs in machine learning. Tensors are multi-dimensional arrays that represent data in a format that models can understand. They are the backbone of neural networks, enabling efficient computation and data manipulation.
Moreover, while pre-trained models offer a solid foundation, they are not a one-size-fits-all solution. Developers must be prepared to fine-tune models for specific tasks or datasets. This process can involve retraining the model on a smaller, domain-specific dataset to improve performance.
In conclusion, the integration of pre-trained neural networks into Java projects is a game-changer. It empowers developers to harness the power of advanced machine learning techniques without the steep learning curve. By leveraging existing models, they can focus on innovation and creativity, driving their projects forward. As technology continues to evolve, the ability to adapt and integrate these tools will be crucial for success in the ever-competitive landscape of software development.
2.
Download the Model: Obtain a pre-trained NER model, such as the one from DeepPavlov.
3. Write the Code: Implement the logic to load the model, tokenize your input text, and run the model to get predictions.
Here’s a snippet to get you started:
```java
import ai.onnxruntime.*;
import java.util.*;
public class NERExample {
public static void main(String[] args) throws OrtException {
String modelPath = "models/deeppavlov_ner_onnx_model.onnx";
OrtEnvironment env = OrtEnvironment.getEnvironment();
OrtSession session = env.createSession(modelPath, new OrtSession.SessionOptions());
String text = "John Doe works at TechCorp and lives in New York.";
String[] tokens = tokenize(text);
long[][] inputIds = prepareInputIds(tokens);
long[][] attentionMask = prepareAttentionMask(tokens);
OrtSession.Result result = session.run(Map.of("input_ids", createTensor(env, inputIds), "attention_mask", createTensor(env, attentionMask)));
processResults(result);
}
// Additional methods for tokenization, input preparation, and result processing
}
```
This code snippet is a mere starting point. It showcases how to load a model and prepare input data. The real magic happens in the details—tokenization, attention masks, and interpreting the model's output.
The advantages of using pre-trained models are clear. They save time, enhance accuracy, and allow developers to focus on higher-level tasks rather than getting bogged down in the intricacies of model training. With the right tools, integrating these models into Java projects becomes a seamless experience.
However, challenges remain. Developers must understand the underlying data structures, such as tensors, which are essential for processing inputs and outputs in machine learning. Tensors are multi-dimensional arrays that represent data in a format that models can understand. They are the backbone of neural networks, enabling efficient computation and data manipulation.
Moreover, while pre-trained models offer a solid foundation, they are not a one-size-fits-all solution. Developers must be prepared to fine-tune models for specific tasks or datasets. This process can involve retraining the model on a smaller, domain-specific dataset to improve performance.
In conclusion, the integration of pre-trained neural networks into Java projects is a game-changer. It empowers developers to harness the power of advanced machine learning techniques without the steep learning curve. By leveraging existing models, they can focus on innovation and creativity, driving their projects forward. As technology continues to evolve, the ability to adapt and integrate these tools will be crucial for success in the ever-competitive landscape of software development.
3.
Write the Code: Implement the logic to load the model, tokenize your input text, and run the model to get predictions.
Here’s a snippet to get you started:
```java
import ai.onnxruntime.*;
import java.util.*;
public class NERExample {
public static void main(String[] args) throws OrtException {
String modelPath = "models/deeppavlov_ner_onnx_model.onnx";
OrtEnvironment env = OrtEnvironment.getEnvironment();
OrtSession session = env.createSession(modelPath, new OrtSession.SessionOptions());
String text = "John Doe works at TechCorp and lives in New York.";
String[] tokens = tokenize(text);
long[][] inputIds = prepareInputIds(tokens);
long[][] attentionMask = prepareAttentionMask(tokens);
OrtSession.Result result = session.run(Map.of("input_ids", createTensor(env, inputIds), "attention_mask", createTensor(env, attentionMask)));
processResults(result);
}
// Additional methods for tokenization, input preparation, and result processing
}
```
This code snippet is a mere starting point. It showcases how to load a model and prepare input data. The real magic happens in the details—tokenization, attention masks, and interpreting the model's output.
The advantages of using pre-trained models are clear. They save time, enhance accuracy, and allow developers to focus on higher-level tasks rather than getting bogged down in the intricacies of model training. With the right tools, integrating these models into Java projects becomes a seamless experience.
However, challenges remain. Developers must understand the underlying data structures, such as tensors, which are essential for processing inputs and outputs in machine learning. Tensors are multi-dimensional arrays that represent data in a format that models can understand. They are the backbone of neural networks, enabling efficient computation and data manipulation.
Moreover, while pre-trained models offer a solid foundation, they are not a one-size-fits-all solution. Developers must be prepared to fine-tune models for specific tasks or datasets. This process can involve retraining the model on a smaller, domain-specific dataset to improve performance.
In conclusion, the integration of pre-trained neural networks into Java projects is a game-changer. It empowers developers to harness the power of advanced machine learning techniques without the steep learning curve. By leveraging existing models, they can focus on innovation and creativity, driving their projects forward. As technology continues to evolve, the ability to adapt and integrate these tools will be crucial for success in the ever-competitive landscape of software development.
Here’s a snippet to get you started:
```java
import ai.onnxruntime.*;
import java.util.*;
public class NERExample {
public static void main(String[] args) throws OrtException {
String modelPath = "models/deeppavlov_ner_onnx_model.onnx";
OrtEnvironment env = OrtEnvironment.getEnvironment();
OrtSession session = env.createSession(modelPath, new OrtSession.SessionOptions());
String text = "John Doe works at TechCorp and lives in New York.";
String[] tokens = tokenize(text);
long[][] inputIds = prepareInputIds(tokens);
long[][] attentionMask = prepareAttentionMask(tokens);
OrtSession.Result result = session.run(Map.of("input_ids", createTensor(env, inputIds), "attention_mask", createTensor(env, attentionMask)));
processResults(result);
}
// Additional methods for tokenization, input preparation, and result processing
}
```
This code snippet is a mere starting point. It showcases how to load a model and prepare input data. The real magic happens in the details—tokenization, attention masks, and interpreting the model's output.
The advantages of using pre-trained models are clear. They save time, enhance accuracy, and allow developers to focus on higher-level tasks rather than getting bogged down in the intricacies of model training. With the right tools, integrating these models into Java projects becomes a seamless experience.
However, challenges remain. Developers must understand the underlying data structures, such as tensors, which are essential for processing inputs and outputs in machine learning. Tensors are multi-dimensional arrays that represent data in a format that models can understand. They are the backbone of neural networks, enabling efficient computation and data manipulation.
Moreover, while pre-trained models offer a solid foundation, they are not a one-size-fits-all solution. Developers must be prepared to fine-tune models for specific tasks or datasets. This process can involve retraining the model on a smaller, domain-specific dataset to improve performance.
In conclusion, the integration of pre-trained neural networks into Java projects is a game-changer. It empowers developers to harness the power of advanced machine learning techniques without the steep learning curve. By leveraging existing models, they can focus on innovation and creativity, driving their projects forward. As technology continues to evolve, the ability to adapt and integrate these tools will be crucial for success in the ever-competitive landscape of software development.