Navigating Django Migrations: A Guide for Developers
December 14, 2024, 12:14 am
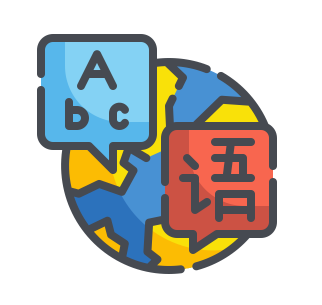
Location: India, Madhya Pradesh, Mohali
Employees: 11-50
Founded date: 2003
Total raised: $600K
Django migrations are like the tide—constant, necessary, and sometimes unpredictable. They shape the landscape of your database, ensuring that your models evolve without losing data. But for many developers, especially those new to Django, migrations can feel like a maze. This guide will illuminate the path, helping you avoid common pitfalls and master the art of migrations.
Migrations are Django's way of propagating changes you make to your models into the database schema. Think of them as blueprints. When you alter a model, Django generates a migration file that describes the changes. This file is a roadmap for your database, detailing how to transform its structure.
But beware! Migrations can lead to confusion, especially when working in teams or across different environments. A small change in one branch can create a ripple effect, causing errors in another.
One of the most frequent issues arises when developers merge branches with differing migration histories. Imagine two paths converging at a fork in the road. If one path has a migration that the other lacks, you might find yourself facing a `FieldDoesNotExist` error. This happens when a migration tries to alter a field that doesn't exist in the current environment.
To navigate this, you can use the `--fake` option. This command tells Django to mark a migration as applied without actually executing it. It’s like signing a document without reading it—useful, but proceed with caution.
Data migrations are a powerful tool often overlooked. They allow you to manipulate data during the migration process. For instance, if you need to import data from a file, a data migration can automate this task.
Creating a data migration involves writing a function that performs the desired operations. This function is then linked to a migration file. It’s essential to ensure that your data migration is idempotent—meaning running it multiple times won’t create duplicates or errors.
For example, if you’re importing a list of apartments from a file, use `get_or_create` instead of `create`. This ensures that if the apartment already exists, it won’t be created again, preventing duplicates.
Before diving into migrations, it’s wise to test the waters. Django offers a `--dry-run` option, allowing you to see what migrations will be applied without actually executing them. This is invaluable for spotting potential issues before they become headaches.
For example, running `python manage.py migrate --plan` will show you the planned operations. It’s like previewing a movie before buying a ticket—better to know what you’re in for.
In the world of Python development, managing dependencies is crucial. Virtual environments are your best friends here. They isolate project dependencies, preventing conflicts.
Creating a virtual environment is straightforward. Use the command `python -m venv venv` to create one. Activate it with `source venv/bin/activate`. Once activated, any packages you install will be confined to this environment, keeping your global Python installation clean.
Package managers like `pip` and `poetry` simplify dependency management. With `pip`, you can install packages using `pip install package_name`. To keep track of your dependencies, maintain a `requirements.txt` file. This file lists all necessary packages, making it easy to replicate your environment elsewhere.
On the other hand, `poetry` offers a more robust solution for complex projects. It manages dependencies and creates a `pyproject.toml` file, which serves as a comprehensive guide to your project’s requirements.
1.Keep Migrations Small
Understanding Migrations
Migrations are Django's way of propagating changes you make to your models into the database schema. Think of them as blueprints. When you alter a model, Django generates a migration file that describes the changes. This file is a roadmap for your database, detailing how to transform its structure.
But beware! Migrations can lead to confusion, especially when working in teams or across different environments. A small change in one branch can create a ripple effect, causing errors in another.
Common Migration Pitfalls
One of the most frequent issues arises when developers merge branches with differing migration histories. Imagine two paths converging at a fork in the road. If one path has a migration that the other lacks, you might find yourself facing a `FieldDoesNotExist` error. This happens when a migration tries to alter a field that doesn't exist in the current environment.
To navigate this, you can use the `--fake` option. This command tells Django to mark a migration as applied without actually executing it. It’s like signing a document without reading it—useful, but proceed with caution.
Data Migrations: The Hidden Gems
Data migrations are a powerful tool often overlooked. They allow you to manipulate data during the migration process. For instance, if you need to import data from a file, a data migration can automate this task.
Creating a data migration involves writing a function that performs the desired operations. This function is then linked to a migration file. It’s essential to ensure that your data migration is idempotent—meaning running it multiple times won’t create duplicates or errors.
For example, if you’re importing a list of apartments from a file, use `get_or_create` instead of `create`. This ensures that if the apartment already exists, it won’t be created again, preventing duplicates.
Dry Runs: Testing the Waters
Before diving into migrations, it’s wise to test the waters. Django offers a `--dry-run` option, allowing you to see what migrations will be applied without actually executing them. This is invaluable for spotting potential issues before they become headaches.
For example, running `python manage.py migrate --plan` will show you the planned operations. It’s like previewing a movie before buying a ticket—better to know what you’re in for.
Managing Virtual Environments
In the world of Python development, managing dependencies is crucial. Virtual environments are your best friends here. They isolate project dependencies, preventing conflicts.
Creating a virtual environment is straightforward. Use the command `python -m venv venv` to create one. Activate it with `source venv/bin/activate`. Once activated, any packages you install will be confined to this environment, keeping your global Python installation clean.
Using Package Managers
Package managers like `pip` and `poetry` simplify dependency management. With `pip`, you can install packages using `pip install package_name`. To keep track of your dependencies, maintain a `requirements.txt` file. This file lists all necessary packages, making it easy to replicate your environment elsewhere.
On the other hand, `poetry` offers a more robust solution for complex projects. It manages dependencies and creates a `pyproject.toml` file, which serves as a comprehensive guide to your project’s requirements.
Best Practices for Migrations
1.
Keep Migrations Small: Large migrations can be daunting. Break them into smaller, manageable pieces. This makes it easier to troubleshoot and understand.
2. Use Descriptive Names: When creating migrations, use clear, descriptive names. This helps you and your team understand the purpose of each migration at a glance.
3. Test Migrations Locally: Before applying migrations in production, test them in a local environment. This can save you from unexpected surprises.
4. Document Your Changes: Maintain a changelog for your migrations. Documenting what each migration does can be a lifesaver for future reference.
5. Stay Consistent: Ensure that all team members follow the same migration practices. Consistency reduces confusion and errors.
Conclusion
Django migrations are a powerful feature that, when understood, can streamline your development process. They are the backbone of your database structure, ensuring that your application evolves smoothly. By avoiding common pitfalls, leveraging data migrations, and managing your environment effectively, you can navigate the complexities of Django with confidence.
Remember, migrations are not just a technical necessity; they are a vital part of your development journey. Embrace them, and they will serve you well.
2.
Use Descriptive Names: When creating migrations, use clear, descriptive names. This helps you and your team understand the purpose of each migration at a glance.
3. Test Migrations Locally: Before applying migrations in production, test them in a local environment. This can save you from unexpected surprises.
4. Document Your Changes: Maintain a changelog for your migrations. Documenting what each migration does can be a lifesaver for future reference.
5. Stay Consistent: Ensure that all team members follow the same migration practices. Consistency reduces confusion and errors.
Conclusion
Django migrations are a powerful feature that, when understood, can streamline your development process. They are the backbone of your database structure, ensuring that your application evolves smoothly. By avoiding common pitfalls, leveraging data migrations, and managing your environment effectively, you can navigate the complexities of Django with confidence.
Remember, migrations are not just a technical necessity; they are a vital part of your development journey. Embrace them, and they will serve you well.
3.
Test Migrations Locally: Before applying migrations in production, test them in a local environment. This can save you from unexpected surprises.
4. Document Your Changes: Maintain a changelog for your migrations. Documenting what each migration does can be a lifesaver for future reference.
5. Stay Consistent: Ensure that all team members follow the same migration practices. Consistency reduces confusion and errors.
Conclusion
Django migrations are a powerful feature that, when understood, can streamline your development process. They are the backbone of your database structure, ensuring that your application evolves smoothly. By avoiding common pitfalls, leveraging data migrations, and managing your environment effectively, you can navigate the complexities of Django with confidence.
Remember, migrations are not just a technical necessity; they are a vital part of your development journey. Embrace them, and they will serve you well.
4.
Document Your Changes: Maintain a changelog for your migrations. Documenting what each migration does can be a lifesaver for future reference.
5. Stay Consistent: Ensure that all team members follow the same migration practices. Consistency reduces confusion and errors.
Conclusion
Django migrations are a powerful feature that, when understood, can streamline your development process. They are the backbone of your database structure, ensuring that your application evolves smoothly. By avoiding common pitfalls, leveraging data migrations, and managing your environment effectively, you can navigate the complexities of Django with confidence.
Remember, migrations are not just a technical necessity; they are a vital part of your development journey. Embrace them, and they will serve you well.
5.
Stay Consistent: Ensure that all team members follow the same migration practices. Consistency reduces confusion and errors.
Conclusion
Django migrations are a powerful feature that, when understood, can streamline your development process. They are the backbone of your database structure, ensuring that your application evolves smoothly. By avoiding common pitfalls, leveraging data migrations, and managing your environment effectively, you can navigate the complexities of Django with confidence.
Remember, migrations are not just a technical necessity; they are a vital part of your development journey. Embrace them, and they will serve you well.
Conclusion
Django migrations are a powerful feature that, when understood, can streamline your development process. They are the backbone of your database structure, ensuring that your application evolves smoothly. By avoiding common pitfalls, leveraging data migrations, and managing your environment effectively, you can navigate the complexities of Django with confidence.
Remember, migrations are not just a technical necessity; they are a vital part of your development journey. Embrace them, and they will serve you well.