The Power of ResultTransformer in Symfony Projects
December 12, 2024, 10:58 am
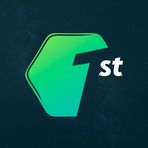
Location: Russia, Moscow Oblast, Сколково
Employees: 51-200
Founded date: 2002
In the world of Symfony, developers often find themselves navigating a labyrinth of data handling. The framework, with its robust set of tools, can feel overwhelming. Yet, within this complexity lies a gem: ResultTransformer. This technique can streamline data transformation, making life easier for developers.
Imagine a factory. Each machine has a specific role. Some machines create raw materials, while others assemble final products. In Symfony, data often needs to be transformed from one format to another. ResultTransformer acts like a conveyor belt, efficiently moving data from one stage to the next without unnecessary manual labor.
### Understanding DTOs
At the heart of this discussion is the Data Transfer Object (DTO). Think of a DTO as a neatly packed suitcase. It holds only what you need for a specific journey. In Symfony, DTOs encapsulate data, making it easier to transport between layers of an application.
For instance, consider a simple UserDTO. It might contain fields like id, name, and email. These fields are essential, just like clothes and toiletries are for a trip. The challenge arises when developers must manually create these DTOs from raw data fetched from the database. This is where ResultTransformer shines.
### The Traditional Approach
Traditionally, developers might fetch data from a repository and manually map it to a DTO. This process can be tedious. Imagine assembling a puzzle without a picture. You know the pieces fit together, but it takes time and effort to see the final image.
Here’s a common approach:
```php
class UserRepository {
public function findAllUsersAsDTO(): array {
$users = $this->findAll();
$userDTOs = [];
foreach ($users as $user) {
$userDTO = new UserDTO($user->getId(), $user->getName(), $user->getEmail());
$userDTOs[] = $userDTO;
}
return $userDTOs;
}
}
```
This method works, but it’s cumbersome. It’s like using a hammer to drive in a screw.
### Enter ResultTransformer
Now, let’s introduce ResultTransformer. This approach allows developers to delegate the heavy lifting to the framework. Instead of manually creating DTOs, you can instruct Doctrine to do it for you.
Here’s how it looks:
```php
class UserRepository {
public function findAllUsersAsDTO(): array {
$query = $this->getEntityManager()->createQuery(
'SELECT NEW App\DTO\User\UserDTO(u.id, u.name, u.email) FROM App\Entity\User u'
);
return $query->getResult();
}
}
```
With this method, you simply tell Doctrine what you want. It’s like ordering a meal at a restaurant. You specify your dish, and the chef prepares it for you. The ResultTransformer simplifies the process, reducing the need for repetitive code.
### The Nuances of ResultTransformer
However, using ResultTransformer isn’t without its challenges. Developers must be cautious. While it streamlines the process, it can also blur the lines between different layers of the application.
For instance, if you rely too heavily on native queries, you might lose the flexibility that comes with using a query builder. To mitigate this, you can create a dedicated DtoResultTransformer class. This class can handle the transformation without tying your repository to specific queries.
```php
class DtoResultTransformer {
public function transform(array $results, string $dtoClass): array {
return array_map(function ($row) use ($dtoClass) {
return new $dtoClass(...array_values($row));
}, $results);
}
}
```
By decoupling the transformation logic, you maintain a clean architecture. It’s like having a separate team for logistics in a factory. They ensure that materials flow smoothly without disrupting production.
### Advanced Techniques
For those who crave efficiency, consider using PHP’s Reflection API. This allows you to automatically determine the required fields for your DTO. It’s like having a smart assistant who knows exactly what you need for your trip without you having to list it out.
```php
public function getRequiredFields(string $dtoClass): array {
$reflection = new ReflectionClass($dtoClass);
$constructor = $reflection->getConstructor();
$parameters = $constructor ? $constructor->getParameters() : [];
return array_map(fn($param) => $param->getName(), $parameters);
}
```
This method reduces manual intervention, making your code cleaner and more maintainable.
### When to Use ResultTransformer
While ResultTransformer is powerful, it’s not a one-size-fits-all solution. It’s best suited for specific scenarios where data aggregation or complex transformations are required. For simpler tasks, traditional methods may suffice.
Think of it as a Swiss Army knife. It’s handy for many tasks, but sometimes a simple screwdriver will do the job just fine.
### Conclusion
In conclusion, ResultTransformer is a valuable tool in the Symfony developer’s toolkit. It simplifies the process of data transformation, allowing developers to focus on building features rather than getting bogged down in repetitive tasks.
However, like any tool, it should be used judiciously. Understand when to deploy it and when to stick with traditional methods. By doing so, you’ll navigate the complexities of Symfony with ease, turning what could be a daunting task into a smooth journey.
Embrace the power of ResultTransformer, and watch your development process transform into a well-oiled machine.
Imagine a factory. Each machine has a specific role. Some machines create raw materials, while others assemble final products. In Symfony, data often needs to be transformed from one format to another. ResultTransformer acts like a conveyor belt, efficiently moving data from one stage to the next without unnecessary manual labor.
### Understanding DTOs
At the heart of this discussion is the Data Transfer Object (DTO). Think of a DTO as a neatly packed suitcase. It holds only what you need for a specific journey. In Symfony, DTOs encapsulate data, making it easier to transport between layers of an application.
For instance, consider a simple UserDTO. It might contain fields like id, name, and email. These fields are essential, just like clothes and toiletries are for a trip. The challenge arises when developers must manually create these DTOs from raw data fetched from the database. This is where ResultTransformer shines.
### The Traditional Approach
Traditionally, developers might fetch data from a repository and manually map it to a DTO. This process can be tedious. Imagine assembling a puzzle without a picture. You know the pieces fit together, but it takes time and effort to see the final image.
Here’s a common approach:
```php
class UserRepository {
public function findAllUsersAsDTO(): array {
$users = $this->findAll();
$userDTOs = [];
foreach ($users as $user) {
$userDTO = new UserDTO($user->getId(), $user->getName(), $user->getEmail());
$userDTOs[] = $userDTO;
}
return $userDTOs;
}
}
```
This method works, but it’s cumbersome. It’s like using a hammer to drive in a screw.
### Enter ResultTransformer
Now, let’s introduce ResultTransformer. This approach allows developers to delegate the heavy lifting to the framework. Instead of manually creating DTOs, you can instruct Doctrine to do it for you.
Here’s how it looks:
```php
class UserRepository {
public function findAllUsersAsDTO(): array {
$query = $this->getEntityManager()->createQuery(
'SELECT NEW App\DTO\User\UserDTO(u.id, u.name, u.email) FROM App\Entity\User u'
);
return $query->getResult();
}
}
```
With this method, you simply tell Doctrine what you want. It’s like ordering a meal at a restaurant. You specify your dish, and the chef prepares it for you. The ResultTransformer simplifies the process, reducing the need for repetitive code.
### The Nuances of ResultTransformer
However, using ResultTransformer isn’t without its challenges. Developers must be cautious. While it streamlines the process, it can also blur the lines between different layers of the application.
For instance, if you rely too heavily on native queries, you might lose the flexibility that comes with using a query builder. To mitigate this, you can create a dedicated DtoResultTransformer class. This class can handle the transformation without tying your repository to specific queries.
```php
class DtoResultTransformer {
public function transform(array $results, string $dtoClass): array {
return array_map(function ($row) use ($dtoClass) {
return new $dtoClass(...array_values($row));
}, $results);
}
}
```
By decoupling the transformation logic, you maintain a clean architecture. It’s like having a separate team for logistics in a factory. They ensure that materials flow smoothly without disrupting production.
### Advanced Techniques
For those who crave efficiency, consider using PHP’s Reflection API. This allows you to automatically determine the required fields for your DTO. It’s like having a smart assistant who knows exactly what you need for your trip without you having to list it out.
```php
public function getRequiredFields(string $dtoClass): array {
$reflection = new ReflectionClass($dtoClass);
$constructor = $reflection->getConstructor();
$parameters = $constructor ? $constructor->getParameters() : [];
return array_map(fn($param) => $param->getName(), $parameters);
}
```
This method reduces manual intervention, making your code cleaner and more maintainable.
### When to Use ResultTransformer
While ResultTransformer is powerful, it’s not a one-size-fits-all solution. It’s best suited for specific scenarios where data aggregation or complex transformations are required. For simpler tasks, traditional methods may suffice.
Think of it as a Swiss Army knife. It’s handy for many tasks, but sometimes a simple screwdriver will do the job just fine.
### Conclusion
In conclusion, ResultTransformer is a valuable tool in the Symfony developer’s toolkit. It simplifies the process of data transformation, allowing developers to focus on building features rather than getting bogged down in repetitive tasks.
However, like any tool, it should be used judiciously. Understand when to deploy it and when to stick with traditional methods. By doing so, you’ll navigate the complexities of Symfony with ease, turning what could be a daunting task into a smooth journey.
Embrace the power of ResultTransformer, and watch your development process transform into a well-oiled machine.