Building Your Own Voice Assistant with Raspberry Pi and Chat API
November 25, 2024, 6:15 am
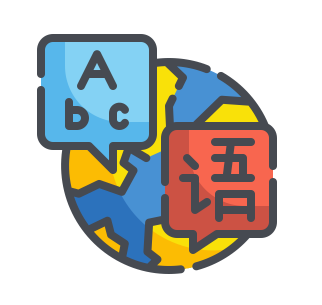
Location: India, Madhya Pradesh, Mohali
Employees: 11-50
Founded date: 2003
Total raised: $600K
In the age of smart technology, voice assistants have become ubiquitous. They are the silent helpers in our homes, responding to our queries and managing our tasks. But what if you could create your own? A voice assistant tailored to your needs, built from scratch. Enter the world of Raspberry Pi and Chat API. This guide will walk you through the process of building a personalized voice assistant that can rival the likes of Google Assistant and Amazon Echo.
Imagine a tiny computer, the size of a credit card. That’s Raspberry Pi. It’s a powerhouse of potential. With it, you can harness the capabilities of artificial intelligence and create something unique. The Chat API serves as the brain of your assistant, processing language and generating responses. Together, they form a dynamic duo.
Before diving into the technical details, let’s set the stage. The first step is to gather your tools. You’ll need a Raspberry Pi, a microphone, and a few software libraries. These libraries will allow your assistant to recognize speech, process language, and convert text to speech. Here’s a quick list of what you need to install:
- `pyaudio`: For audio input.
- `speechrecognition`: To recognize spoken words.
- `transformers`: For natural language processing.
- `openai`: To interact with the Chat API.
Install these libraries using Python’s package manager, pip. This is your toolkit for building a voice assistant.
Next, let’s set up the Raspberry Pi. Follow the official documentation to install the operating system. Once that’s done, connect your microphone, keyboard, and mouse. These peripherals will allow you to interact with your assistant. Choose high-quality devices for better performance.
Power is crucial. Use a reliable power supply to avoid instability. Raspberry Pi requires a steady five volts to function optimally. Think of it as the lifeblood of your project.
Now comes the fun part: coding. You’ll implement the logic that makes your assistant work. Start by setting up the activation word detection. This is the phrase that wakes your assistant. Use the `speechrecognition` library to listen for your chosen activation phrase. Here’s a simple snippet:
```python
import speech_recognition as sr
r = sr.Recognizer()
with sr.Microphone() as source:
print("Listening...")
audio = r.listen(source)
try:
text = r.recognize_google(audio)
if "Hey Assistant" in text:
print("Activation word detected!")
except sr.UnknownValueError:
print("Could not understand audio")
except sr.RequestError as e:
print(f"Error with the speech recognition service; {e}")
```
This code listens for your activation phrase. If it hears it, your assistant is ready to respond.
Once activated, your assistant needs to understand commands. Integrate a speech recognition system to convert audio into text. This is where the magic happens. Use the Google Speech-to-Text API for accurate transcription. Here’s how:
```python
audio = r.listen(source)
try:
text = r.recognize_google(audio)
print(f"Recognized text: {text}")
except sr.UnknownValueError:
print("Could not understand audio")
except sr.RequestError as e:
print(f"Error with the speech recognition service; {e}")
```
With the text in hand, it’s time to process it. Send the recognized text to the Chat API. This API will analyze the input and generate a response. Here’s a simple function to get a response:
```python
import openai
openai.api_key = "YOUR_API_KEY"
def get_chat_response(user_input):
response = openai.Completion.create(
engine="text-davinci-003",
prompt=user_input,
max_tokens=150,
n=1,
stop=None,
temperature=0.7,
)
return response.choices[0].text.strip()
```
This function takes user input and returns a response from the Chat API. It’s like having a conversation with a knowledgeable friend.
Now, let’s make your assistant speak. Use a text-to-speech library like gTTS (Google Text-to-Speech) to convert the API’s response into audio. Here’s how:
```python
from gtts import gTTS
import os
def speak(text):
tts = gTTS(text=text, lang='en')
tts.save("output.mp3")
os.system("mpg321 output.mp3")
```
This function takes the text response and converts it into speech. Your assistant can now communicate back to you.
After coding, it’s time to test your creation. Run various scenarios to ensure it responds accurately. Check the response time and clarity of speech. This phase is crucial. It’s where you refine your assistant, making it more reliable and user-friendly.
Building your own voice assistant is an exciting journey. It’s a blend of creativity and technology. With Raspberry Pi and Chat API, you can create a personalized assistant that meets your needs. Follow the steps outlined here, and you’ll have a functional voice assistant in no time. Embrace the challenge, and let your imagination soar. The future of personal technology is in your hands.
Imagine a tiny computer, the size of a credit card. That’s Raspberry Pi. It’s a powerhouse of potential. With it, you can harness the capabilities of artificial intelligence and create something unique. The Chat API serves as the brain of your assistant, processing language and generating responses. Together, they form a dynamic duo.
Getting Started
Before diving into the technical details, let’s set the stage. The first step is to gather your tools. You’ll need a Raspberry Pi, a microphone, and a few software libraries. These libraries will allow your assistant to recognize speech, process language, and convert text to speech. Here’s a quick list of what you need to install:
- `pyaudio`: For audio input.
- `speechrecognition`: To recognize spoken words.
- `transformers`: For natural language processing.
- `openai`: To interact with the Chat API.
Install these libraries using Python’s package manager, pip. This is your toolkit for building a voice assistant.
Setting Up Raspberry Pi
Next, let’s set up the Raspberry Pi. Follow the official documentation to install the operating system. Once that’s done, connect your microphone, keyboard, and mouse. These peripherals will allow you to interact with your assistant. Choose high-quality devices for better performance.
Power is crucial. Use a reliable power supply to avoid instability. Raspberry Pi requires a steady five volts to function optimally. Think of it as the lifeblood of your project.
Writing the Code
Now comes the fun part: coding. You’ll implement the logic that makes your assistant work. Start by setting up the activation word detection. This is the phrase that wakes your assistant. Use the `speechrecognition` library to listen for your chosen activation phrase. Here’s a simple snippet:
```python
import speech_recognition as sr
r = sr.Recognizer()
with sr.Microphone() as source:
print("Listening...")
audio = r.listen(source)
try:
text = r.recognize_google(audio)
if "Hey Assistant" in text:
print("Activation word detected!")
except sr.UnknownValueError:
print("Could not understand audio")
except sr.RequestError as e:
print(f"Error with the speech recognition service; {e}")
```
This code listens for your activation phrase. If it hears it, your assistant is ready to respond.
Speech Recognition and Processing
Once activated, your assistant needs to understand commands. Integrate a speech recognition system to convert audio into text. This is where the magic happens. Use the Google Speech-to-Text API for accurate transcription. Here’s how:
```python
audio = r.listen(source)
try:
text = r.recognize_google(audio)
print(f"Recognized text: {text}")
except sr.UnknownValueError:
print("Could not understand audio")
except sr.RequestError as e:
print(f"Error with the speech recognition service; {e}")
```
With the text in hand, it’s time to process it. Send the recognized text to the Chat API. This API will analyze the input and generate a response. Here’s a simple function to get a response:
```python
import openai
openai.api_key = "YOUR_API_KEY"
def get_chat_response(user_input):
response = openai.Completion.create(
engine="text-davinci-003",
prompt=user_input,
max_tokens=150,
n=1,
stop=None,
temperature=0.7,
)
return response.choices[0].text.strip()
```
This function takes user input and returns a response from the Chat API. It’s like having a conversation with a knowledgeable friend.
Text-to-Speech Conversion
Now, let’s make your assistant speak. Use a text-to-speech library like gTTS (Google Text-to-Speech) to convert the API’s response into audio. Here’s how:
```python
from gtts import gTTS
import os
def speak(text):
tts = gTTS(text=text, lang='en')
tts.save("output.mp3")
os.system("mpg321 output.mp3")
```
This function takes the text response and converts it into speech. Your assistant can now communicate back to you.
Testing and Optimization
After coding, it’s time to test your creation. Run various scenarios to ensure it responds accurately. Check the response time and clarity of speech. This phase is crucial. It’s where you refine your assistant, making it more reliable and user-friendly.
Conclusion
Building your own voice assistant is an exciting journey. It’s a blend of creativity and technology. With Raspberry Pi and Chat API, you can create a personalized assistant that meets your needs. Follow the steps outlined here, and you’ll have a functional voice assistant in no time. Embrace the challenge, and let your imagination soar. The future of personal technology is in your hands.