The Art of Caching and Versioning in Microservices
November 21, 2024, 5:22 pm
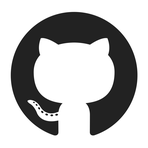
Location: United States, California, San Francisco
Employees: 1001-5000
Founded date: 2008
Total raised: $350M
In the world of microservices, speed and reliability are paramount. Imagine a bustling city where every car represents a service. Some cars zoom by, while others crawl at a snail's pace. The key to transforming sluggish services into speedy ones lies in caching and versioning. These two concepts can turn your microservices architecture from a clunky old bus into a sleek sports car.
### Caching: The Fast Lane
Caching is like a shortcut in a busy city. It stores frequently accessed data, reducing the need for repeated trips to the database or external APIs. This not only speeds up response times but also alleviates pressure on your services.
Every time a service makes a call to another service, it’s like sending a letter across town. There’s a delay. Caching allows you to keep a copy of that letter on hand, so you don’t have to send it again. This is especially crucial when dealing with high traffic or repeated requests for the same data.
FeignClient is a powerful tool in the Spring ecosystem. It simplifies communication between microservices, but it can also introduce latency. Each call to a FeignClient is a network request, which can slow down your application. By integrating caching, you can significantly reduce these delays.
To implement caching with FeignClient, you can use Caffeine Cache, a high-performance caching library. Here’s how to set it up:
1.Add Dependencies
### Caching: The Fast Lane
Caching is like a shortcut in a busy city. It stores frequently accessed data, reducing the need for repeated trips to the database or external APIs. This not only speeds up response times but also alleviates pressure on your services.
Why Caching Matters
Every time a service makes a call to another service, it’s like sending a letter across town. There’s a delay. Caching allows you to keep a copy of that letter on hand, so you don’t have to send it again. This is especially crucial when dealing with high traffic or repeated requests for the same data.
Implementing Caching with FeignClient
FeignClient is a powerful tool in the Spring ecosystem. It simplifies communication between microservices, but it can also introduce latency. Each call to a FeignClient is a network request, which can slow down your application. By integrating caching, you can significantly reduce these delays.
To implement caching with FeignClient, you can use Caffeine Cache, a high-performance caching library. Here’s how to set it up:
1.
Add Dependencies: Start by including the necessary dependencies in your `build.gradle` file. This includes Spring Boot, Spring Cloud OpenFeign, and Caffeine.
2. Create a FeignClient: Define your FeignClient interface. This is where you specify the external API you want to communicate with.
3. Enable Caching: Use the `@EnableCaching` annotation in your configuration class. This tells Spring to manage caching for you.
4. Cache the Results: Annotate your service methods with `@Cacheable`. This tells Spring to check the cache before making a network call. If the data is in the cache, it returns it immediately. If not, it fetches the data and stores it in the cache for future requests.
By following these steps, you can transform your service into a high-speed machine, capable of handling requests with ease.
### Versioning: The Roadmap to Stability
While caching speeds up your services, versioning ensures stability. Imagine navigating a city with constantly changing road signs. Without clear directions, drivers would be lost. Versioning provides that clarity, allowing different parts of your application to evolve independently.
Why Versioning is Essential
Without versioning, deploying new features can be risky. If the frontend and backend are tightly coupled, a change in one can break the other. Versioning allows you to introduce new features without disrupting existing functionality. It’s like having multiple lanes on a highway; each lane can evolve at its own pace.
Implementing Endpoint Versioning
There are several strategies for versioning your APIs. Here are a few common approaches:
1. URI Versioning: Include the version number in the URL, such as `/api/v1/resource`. This is straightforward and easy to understand.
2. Query Parameter Versioning: Use a query parameter to specify the version, like `/api/resource?version=1`. This keeps the URL clean but can be less intuitive.
3. Header Versioning: Specify the version in the request header. This keeps the URL unchanged but requires clients to manage headers.
Each method has its pros and cons, but the goal remains the same: to provide a clear contract between the frontend and backend.
### Global Versioning: A Unified Approach
A common challenge with traditional versioning is managing multiple versions across various endpoints. This can lead to confusion and complexity. Global versioning simplifies this by applying a single version number across all endpoints.
How to Implement Global Versioning
1. Single Version Number: Use one version number for the entire API. When any change is made, increment this number. This keeps things simple and consistent.
2. Minimal Code Changes: Strive to keep changes minimal. The goal is to avoid extensive modifications to existing code when introducing new features.
3. Impact on Codebase: Ensure that adding new versions or endpoints has minimal impact on the existing codebase. This helps maintain stability and reduces the risk of introducing bugs.
### Testing: The Safety Net
No implementation is complete without thorough testing. Ensure that your caching and versioning strategies work as intended. Write tests to verify that cached data is returned correctly and that versioned endpoints respond as expected. This is your safety net, catching any issues before they reach production.
### Conclusion: The Journey Ahead
Caching and versioning are not just technical implementations; they are essential strategies for building robust microservices. Caching accelerates your services, while versioning provides stability and flexibility. Together, they create a seamless experience for users and developers alike.
As you embark on your journey to optimize your microservices architecture, remember: speed and stability are your guiding stars. Embrace caching and versioning, and watch your services transform from sluggish to swift. The road ahead may be challenging, but with the right tools, you can navigate it with confidence.
2.
Create a FeignClient: Define your FeignClient interface. This is where you specify the external API you want to communicate with.
3. Enable Caching: Use the `@EnableCaching` annotation in your configuration class. This tells Spring to manage caching for you.
4. Cache the Results: Annotate your service methods with `@Cacheable`. This tells Spring to check the cache before making a network call. If the data is in the cache, it returns it immediately. If not, it fetches the data and stores it in the cache for future requests.
By following these steps, you can transform your service into a high-speed machine, capable of handling requests with ease.
### Versioning: The Roadmap to Stability
While caching speeds up your services, versioning ensures stability. Imagine navigating a city with constantly changing road signs. Without clear directions, drivers would be lost. Versioning provides that clarity, allowing different parts of your application to evolve independently.
Why Versioning is Essential
Without versioning, deploying new features can be risky. If the frontend and backend are tightly coupled, a change in one can break the other. Versioning allows you to introduce new features without disrupting existing functionality. It’s like having multiple lanes on a highway; each lane can evolve at its own pace.
Implementing Endpoint Versioning
There are several strategies for versioning your APIs. Here are a few common approaches:
1. URI Versioning: Include the version number in the URL, such as `/api/v1/resource`. This is straightforward and easy to understand.
2. Query Parameter Versioning: Use a query parameter to specify the version, like `/api/resource?version=1`. This keeps the URL clean but can be less intuitive.
3. Header Versioning: Specify the version in the request header. This keeps the URL unchanged but requires clients to manage headers.
Each method has its pros and cons, but the goal remains the same: to provide a clear contract between the frontend and backend.
### Global Versioning: A Unified Approach
A common challenge with traditional versioning is managing multiple versions across various endpoints. This can lead to confusion and complexity. Global versioning simplifies this by applying a single version number across all endpoints.
How to Implement Global Versioning
1. Single Version Number: Use one version number for the entire API. When any change is made, increment this number. This keeps things simple and consistent.
2. Minimal Code Changes: Strive to keep changes minimal. The goal is to avoid extensive modifications to existing code when introducing new features.
3. Impact on Codebase: Ensure that adding new versions or endpoints has minimal impact on the existing codebase. This helps maintain stability and reduces the risk of introducing bugs.
### Testing: The Safety Net
No implementation is complete without thorough testing. Ensure that your caching and versioning strategies work as intended. Write tests to verify that cached data is returned correctly and that versioned endpoints respond as expected. This is your safety net, catching any issues before they reach production.
### Conclusion: The Journey Ahead
Caching and versioning are not just technical implementations; they are essential strategies for building robust microservices. Caching accelerates your services, while versioning provides stability and flexibility. Together, they create a seamless experience for users and developers alike.
As you embark on your journey to optimize your microservices architecture, remember: speed and stability are your guiding stars. Embrace caching and versioning, and watch your services transform from sluggish to swift. The road ahead may be challenging, but with the right tools, you can navigate it with confidence.
3.
Enable Caching: Use the `@EnableCaching` annotation in your configuration class. This tells Spring to manage caching for you.
4. Cache the Results: Annotate your service methods with `@Cacheable`. This tells Spring to check the cache before making a network call. If the data is in the cache, it returns it immediately. If not, it fetches the data and stores it in the cache for future requests.
By following these steps, you can transform your service into a high-speed machine, capable of handling requests with ease.
### Versioning: The Roadmap to Stability
While caching speeds up your services, versioning ensures stability. Imagine navigating a city with constantly changing road signs. Without clear directions, drivers would be lost. Versioning provides that clarity, allowing different parts of your application to evolve independently.
Why Versioning is Essential
Without versioning, deploying new features can be risky. If the frontend and backend are tightly coupled, a change in one can break the other. Versioning allows you to introduce new features without disrupting existing functionality. It’s like having multiple lanes on a highway; each lane can evolve at its own pace.
Implementing Endpoint Versioning
There are several strategies for versioning your APIs. Here are a few common approaches:
1. URI Versioning: Include the version number in the URL, such as `/api/v1/resource`. This is straightforward and easy to understand.
2. Query Parameter Versioning: Use a query parameter to specify the version, like `/api/resource?version=1`. This keeps the URL clean but can be less intuitive.
3. Header Versioning: Specify the version in the request header. This keeps the URL unchanged but requires clients to manage headers.
Each method has its pros and cons, but the goal remains the same: to provide a clear contract between the frontend and backend.
### Global Versioning: A Unified Approach
A common challenge with traditional versioning is managing multiple versions across various endpoints. This can lead to confusion and complexity. Global versioning simplifies this by applying a single version number across all endpoints.
How to Implement Global Versioning
1. Single Version Number: Use one version number for the entire API. When any change is made, increment this number. This keeps things simple and consistent.
2. Minimal Code Changes: Strive to keep changes minimal. The goal is to avoid extensive modifications to existing code when introducing new features.
3. Impact on Codebase: Ensure that adding new versions or endpoints has minimal impact on the existing codebase. This helps maintain stability and reduces the risk of introducing bugs.
### Testing: The Safety Net
No implementation is complete without thorough testing. Ensure that your caching and versioning strategies work as intended. Write tests to verify that cached data is returned correctly and that versioned endpoints respond as expected. This is your safety net, catching any issues before they reach production.
### Conclusion: The Journey Ahead
Caching and versioning are not just technical implementations; they are essential strategies for building robust microservices. Caching accelerates your services, while versioning provides stability and flexibility. Together, they create a seamless experience for users and developers alike.
As you embark on your journey to optimize your microservices architecture, remember: speed and stability are your guiding stars. Embrace caching and versioning, and watch your services transform from sluggish to swift. The road ahead may be challenging, but with the right tools, you can navigate it with confidence.
4.
Cache the Results: Annotate your service methods with `@Cacheable`. This tells Spring to check the cache before making a network call. If the data is in the cache, it returns it immediately. If not, it fetches the data and stores it in the cache for future requests.
By following these steps, you can transform your service into a high-speed machine, capable of handling requests with ease.
### Versioning: The Roadmap to Stability
While caching speeds up your services, versioning ensures stability. Imagine navigating a city with constantly changing road signs. Without clear directions, drivers would be lost. Versioning provides that clarity, allowing different parts of your application to evolve independently.
Why Versioning is Essential
Without versioning, deploying new features can be risky. If the frontend and backend are tightly coupled, a change in one can break the other. Versioning allows you to introduce new features without disrupting existing functionality. It’s like having multiple lanes on a highway; each lane can evolve at its own pace.
Implementing Endpoint Versioning
There are several strategies for versioning your APIs. Here are a few common approaches:
1. URI Versioning: Include the version number in the URL, such as `/api/v1/resource`. This is straightforward and easy to understand.
2. Query Parameter Versioning: Use a query parameter to specify the version, like `/api/resource?version=1`. This keeps the URL clean but can be less intuitive.
3. Header Versioning: Specify the version in the request header. This keeps the URL unchanged but requires clients to manage headers.
Each method has its pros and cons, but the goal remains the same: to provide a clear contract between the frontend and backend.
### Global Versioning: A Unified Approach
A common challenge with traditional versioning is managing multiple versions across various endpoints. This can lead to confusion and complexity. Global versioning simplifies this by applying a single version number across all endpoints.
How to Implement Global Versioning
1. Single Version Number: Use one version number for the entire API. When any change is made, increment this number. This keeps things simple and consistent.
2. Minimal Code Changes: Strive to keep changes minimal. The goal is to avoid extensive modifications to existing code when introducing new features.
3. Impact on Codebase: Ensure that adding new versions or endpoints has minimal impact on the existing codebase. This helps maintain stability and reduces the risk of introducing bugs.
### Testing: The Safety Net
No implementation is complete without thorough testing. Ensure that your caching and versioning strategies work as intended. Write tests to verify that cached data is returned correctly and that versioned endpoints respond as expected. This is your safety net, catching any issues before they reach production.
### Conclusion: The Journey Ahead
Caching and versioning are not just technical implementations; they are essential strategies for building robust microservices. Caching accelerates your services, while versioning provides stability and flexibility. Together, they create a seamless experience for users and developers alike.
As you embark on your journey to optimize your microservices architecture, remember: speed and stability are your guiding stars. Embrace caching and versioning, and watch your services transform from sluggish to swift. The road ahead may be challenging, but with the right tools, you can navigate it with confidence.
By following these steps, you can transform your service into a high-speed machine, capable of handling requests with ease.
### Versioning: The Roadmap to Stability
While caching speeds up your services, versioning ensures stability. Imagine navigating a city with constantly changing road signs. Without clear directions, drivers would be lost. Versioning provides that clarity, allowing different parts of your application to evolve independently.
Why Versioning is Essential
Without versioning, deploying new features can be risky. If the frontend and backend are tightly coupled, a change in one can break the other. Versioning allows you to introduce new features without disrupting existing functionality. It’s like having multiple lanes on a highway; each lane can evolve at its own pace.
Implementing Endpoint Versioning
There are several strategies for versioning your APIs. Here are a few common approaches:
1.
URI Versioning: Include the version number in the URL, such as `/api/v1/resource`. This is straightforward and easy to understand.
2. Query Parameter Versioning: Use a query parameter to specify the version, like `/api/resource?version=1`. This keeps the URL clean but can be less intuitive.
3. Header Versioning: Specify the version in the request header. This keeps the URL unchanged but requires clients to manage headers.
Each method has its pros and cons, but the goal remains the same: to provide a clear contract between the frontend and backend.
### Global Versioning: A Unified Approach
A common challenge with traditional versioning is managing multiple versions across various endpoints. This can lead to confusion and complexity. Global versioning simplifies this by applying a single version number across all endpoints.
How to Implement Global Versioning
1. Single Version Number: Use one version number for the entire API. When any change is made, increment this number. This keeps things simple and consistent.
2. Minimal Code Changes: Strive to keep changes minimal. The goal is to avoid extensive modifications to existing code when introducing new features.
3. Impact on Codebase: Ensure that adding new versions or endpoints has minimal impact on the existing codebase. This helps maintain stability and reduces the risk of introducing bugs.
### Testing: The Safety Net
No implementation is complete without thorough testing. Ensure that your caching and versioning strategies work as intended. Write tests to verify that cached data is returned correctly and that versioned endpoints respond as expected. This is your safety net, catching any issues before they reach production.
### Conclusion: The Journey Ahead
Caching and versioning are not just technical implementations; they are essential strategies for building robust microservices. Caching accelerates your services, while versioning provides stability and flexibility. Together, they create a seamless experience for users and developers alike.
As you embark on your journey to optimize your microservices architecture, remember: speed and stability are your guiding stars. Embrace caching and versioning, and watch your services transform from sluggish to swift. The road ahead may be challenging, but with the right tools, you can navigate it with confidence.
2.
Query Parameter Versioning: Use a query parameter to specify the version, like `/api/resource?version=1`. This keeps the URL clean but can be less intuitive.
3. Header Versioning: Specify the version in the request header. This keeps the URL unchanged but requires clients to manage headers.
Each method has its pros and cons, but the goal remains the same: to provide a clear contract between the frontend and backend.
### Global Versioning: A Unified Approach
A common challenge with traditional versioning is managing multiple versions across various endpoints. This can lead to confusion and complexity. Global versioning simplifies this by applying a single version number across all endpoints.
How to Implement Global Versioning
1. Single Version Number: Use one version number for the entire API. When any change is made, increment this number. This keeps things simple and consistent.
2. Minimal Code Changes: Strive to keep changes minimal. The goal is to avoid extensive modifications to existing code when introducing new features.
3. Impact on Codebase: Ensure that adding new versions or endpoints has minimal impact on the existing codebase. This helps maintain stability and reduces the risk of introducing bugs.
### Testing: The Safety Net
No implementation is complete without thorough testing. Ensure that your caching and versioning strategies work as intended. Write tests to verify that cached data is returned correctly and that versioned endpoints respond as expected. This is your safety net, catching any issues before they reach production.
### Conclusion: The Journey Ahead
Caching and versioning are not just technical implementations; they are essential strategies for building robust microservices. Caching accelerates your services, while versioning provides stability and flexibility. Together, they create a seamless experience for users and developers alike.
As you embark on your journey to optimize your microservices architecture, remember: speed and stability are your guiding stars. Embrace caching and versioning, and watch your services transform from sluggish to swift. The road ahead may be challenging, but with the right tools, you can navigate it with confidence.
3.
Header Versioning: Specify the version in the request header. This keeps the URL unchanged but requires clients to manage headers.
Each method has its pros and cons, but the goal remains the same: to provide a clear contract between the frontend and backend.
### Global Versioning: A Unified Approach
A common challenge with traditional versioning is managing multiple versions across various endpoints. This can lead to confusion and complexity. Global versioning simplifies this by applying a single version number across all endpoints.
How to Implement Global Versioning
1. Single Version Number: Use one version number for the entire API. When any change is made, increment this number. This keeps things simple and consistent.
2. Minimal Code Changes: Strive to keep changes minimal. The goal is to avoid extensive modifications to existing code when introducing new features.
3. Impact on Codebase: Ensure that adding new versions or endpoints has minimal impact on the existing codebase. This helps maintain stability and reduces the risk of introducing bugs.
### Testing: The Safety Net
No implementation is complete without thorough testing. Ensure that your caching and versioning strategies work as intended. Write tests to verify that cached data is returned correctly and that versioned endpoints respond as expected. This is your safety net, catching any issues before they reach production.
### Conclusion: The Journey Ahead
Caching and versioning are not just technical implementations; they are essential strategies for building robust microservices. Caching accelerates your services, while versioning provides stability and flexibility. Together, they create a seamless experience for users and developers alike.
As you embark on your journey to optimize your microservices architecture, remember: speed and stability are your guiding stars. Embrace caching and versioning, and watch your services transform from sluggish to swift. The road ahead may be challenging, but with the right tools, you can navigate it with confidence.
Each method has its pros and cons, but the goal remains the same: to provide a clear contract between the frontend and backend.
### Global Versioning: A Unified Approach
A common challenge with traditional versioning is managing multiple versions across various endpoints. This can lead to confusion and complexity. Global versioning simplifies this by applying a single version number across all endpoints.
How to Implement Global Versioning
1.
Single Version Number: Use one version number for the entire API. When any change is made, increment this number. This keeps things simple and consistent.
2. Minimal Code Changes: Strive to keep changes minimal. The goal is to avoid extensive modifications to existing code when introducing new features.
3. Impact on Codebase: Ensure that adding new versions or endpoints has minimal impact on the existing codebase. This helps maintain stability and reduces the risk of introducing bugs.
### Testing: The Safety Net
No implementation is complete without thorough testing. Ensure that your caching and versioning strategies work as intended. Write tests to verify that cached data is returned correctly and that versioned endpoints respond as expected. This is your safety net, catching any issues before they reach production.
### Conclusion: The Journey Ahead
Caching and versioning are not just technical implementations; they are essential strategies for building robust microservices. Caching accelerates your services, while versioning provides stability and flexibility. Together, they create a seamless experience for users and developers alike.
As you embark on your journey to optimize your microservices architecture, remember: speed and stability are your guiding stars. Embrace caching and versioning, and watch your services transform from sluggish to swift. The road ahead may be challenging, but with the right tools, you can navigate it with confidence.
2.
Minimal Code Changes: Strive to keep changes minimal. The goal is to avoid extensive modifications to existing code when introducing new features.
3. Impact on Codebase: Ensure that adding new versions or endpoints has minimal impact on the existing codebase. This helps maintain stability and reduces the risk of introducing bugs.
### Testing: The Safety Net
No implementation is complete without thorough testing. Ensure that your caching and versioning strategies work as intended. Write tests to verify that cached data is returned correctly and that versioned endpoints respond as expected. This is your safety net, catching any issues before they reach production.
### Conclusion: The Journey Ahead
Caching and versioning are not just technical implementations; they are essential strategies for building robust microservices. Caching accelerates your services, while versioning provides stability and flexibility. Together, they create a seamless experience for users and developers alike.
As you embark on your journey to optimize your microservices architecture, remember: speed and stability are your guiding stars. Embrace caching and versioning, and watch your services transform from sluggish to swift. The road ahead may be challenging, but with the right tools, you can navigate it with confidence.
3.
Impact on Codebase: Ensure that adding new versions or endpoints has minimal impact on the existing codebase. This helps maintain stability and reduces the risk of introducing bugs.
### Testing: The Safety Net
No implementation is complete without thorough testing. Ensure that your caching and versioning strategies work as intended. Write tests to verify that cached data is returned correctly and that versioned endpoints respond as expected. This is your safety net, catching any issues before they reach production.
### Conclusion: The Journey Ahead
Caching and versioning are not just technical implementations; they are essential strategies for building robust microservices. Caching accelerates your services, while versioning provides stability and flexibility. Together, they create a seamless experience for users and developers alike.
As you embark on your journey to optimize your microservices architecture, remember: speed and stability are your guiding stars. Embrace caching and versioning, and watch your services transform from sluggish to swift. The road ahead may be challenging, but with the right tools, you can navigate it with confidence.
### Testing: The Safety Net
No implementation is complete without thorough testing. Ensure that your caching and versioning strategies work as intended. Write tests to verify that cached data is returned correctly and that versioned endpoints respond as expected. This is your safety net, catching any issues before they reach production.
### Conclusion: The Journey Ahead
Caching and versioning are not just technical implementations; they are essential strategies for building robust microservices. Caching accelerates your services, while versioning provides stability and flexibility. Together, they create a seamless experience for users and developers alike.
As you embark on your journey to optimize your microservices architecture, remember: speed and stability are your guiding stars. Embrace caching and versioning, and watch your services transform from sluggish to swift. The road ahead may be challenging, but with the right tools, you can navigate it with confidence.