Navigating the Maze of Recursive Dependencies in Frontend Development
October 23, 2024, 5:54 am
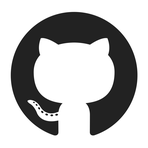
Location: United States, California, San Francisco
Employees: 1001-5000
Founded date: 2008
Total raised: $350M
In the world of frontend development, recursive dependencies can feel like a labyrinth. They twist and turn, creating paths that lead to confusion and errors. Understanding these dependencies is crucial for building robust applications. This article delves into the nature of recursive dependencies, their impact on development, and effective strategies to manage them.
Recursive dependencies occur when modules reference each other, either directly or indirectly. Imagine two friends passing a ball back and forth endlessly. This is what happens in code when Module A imports Module B, and Module B imports Module A. The cycle creates a deadlock, halting the build process. Tools like Webpack or Vite try to resolve these dependencies, but they can stumble upon cycles, leading to incomplete builds or runtime errors.
The symptoms of these issues can be subtle. Tests may fail unexpectedly, citing undefined variables. Users might experience incomplete functionality on the site. The problem is particularly pronounced in microfrontend architectures, where multiple applications coexist. If shared modules differ in version, the entire application can falter. Keeping track of these versions is vital. Tools like nx.dev can help manage dependencies and ensure that microfrontends are updated appropriately.
To mitigate recursive dependencies, developers can adopt a new approach. Extracting common code into a shared library can eliminate direct references between microfrontends. This separation reduces the likelihood of creating cycles. Dynamic imports can also be a lifesaver. By loading modules only when needed, developers can sidestep the pitfalls of circular dependencies. However, this doesn't guarantee that cycles won't occur; it merely lowers the chances.
Consider a simple example of direct recursion:
```javascript
// A.ts
import { B } from './B';
export const A = () => B();
// B.ts
import { A } from './A';
export const B = () => A();
```
In this scenario, both modules are locked in a recursive embrace. However, if neither module utilizes its dependencies, the bundler may exclude them from the final build. This is known as tree-shaking, where unused code is removed.
Indirect recursion can be even trickier. Imagine a chain of modules, each importing the next, eventually leading back to the first. This can create a tangled web that is hard to untangle. The solution lies in dynamic imports, which load modules on demand, preventing simultaneous initialization.
Another effective strategy is to reorganize project structure. Often, developers export everything from an index file, creating hidden dependencies. This can lead to situations where a module tries to access an undefined variable because the initialization order is disrupted. Instead, use direct imports to ensure clarity and prevent cycles.
Tools can assist in identifying recursive dependencies. For instance, eslint-plugin-import can help detect these issues, though it may slow down on larger projects. Using eslint_d can speed up checks. Webpack offers the circular-dependency-plugin, but it struggles with complex scenarios. It's best to run such checks during merge requests rather than as part of the main build process.
Resolving recursive dependencies is essential for maintaining clean, stable code. While bundlers can often navigate these issues, relying on them isn't a long-term solution. During testing, switching from SWC to Babel in a project can expose hidden recursive dependencies. The sideEffects property in package.json can help manage these situations, indicating that modules may have side effects that affect initialization.
In conclusion, understanding and managing recursive dependencies is a vital skill for frontend developers. By adopting best practices, utilizing tools, and reorganizing code structures, developers can navigate the maze of dependencies with confidence. The goal is to create a stable, maintainable codebase that stands the test of time. As the landscape of frontend development evolves, staying ahead of these challenges will ensure success in building robust applications.
Recursive dependencies occur when modules reference each other, either directly or indirectly. Imagine two friends passing a ball back and forth endlessly. This is what happens in code when Module A imports Module B, and Module B imports Module A. The cycle creates a deadlock, halting the build process. Tools like Webpack or Vite try to resolve these dependencies, but they can stumble upon cycles, leading to incomplete builds or runtime errors.
The symptoms of these issues can be subtle. Tests may fail unexpectedly, citing undefined variables. Users might experience incomplete functionality on the site. The problem is particularly pronounced in microfrontend architectures, where multiple applications coexist. If shared modules differ in version, the entire application can falter. Keeping track of these versions is vital. Tools like nx.dev can help manage dependencies and ensure that microfrontends are updated appropriately.
To mitigate recursive dependencies, developers can adopt a new approach. Extracting common code into a shared library can eliminate direct references between microfrontends. This separation reduces the likelihood of creating cycles. Dynamic imports can also be a lifesaver. By loading modules only when needed, developers can sidestep the pitfalls of circular dependencies. However, this doesn't guarantee that cycles won't occur; it merely lowers the chances.
Consider a simple example of direct recursion:
```javascript
// A.ts
import { B } from './B';
export const A = () => B();
// B.ts
import { A } from './A';
export const B = () => A();
```
In this scenario, both modules are locked in a recursive embrace. However, if neither module utilizes its dependencies, the bundler may exclude them from the final build. This is known as tree-shaking, where unused code is removed.
Indirect recursion can be even trickier. Imagine a chain of modules, each importing the next, eventually leading back to the first. This can create a tangled web that is hard to untangle. The solution lies in dynamic imports, which load modules on demand, preventing simultaneous initialization.
Another effective strategy is to reorganize project structure. Often, developers export everything from an index file, creating hidden dependencies. This can lead to situations where a module tries to access an undefined variable because the initialization order is disrupted. Instead, use direct imports to ensure clarity and prevent cycles.
Tools can assist in identifying recursive dependencies. For instance, eslint-plugin-import can help detect these issues, though it may slow down on larger projects. Using eslint_d can speed up checks. Webpack offers the circular-dependency-plugin, but it struggles with complex scenarios. It's best to run such checks during merge requests rather than as part of the main build process.
Resolving recursive dependencies is essential for maintaining clean, stable code. While bundlers can often navigate these issues, relying on them isn't a long-term solution. During testing, switching from SWC to Babel in a project can expose hidden recursive dependencies. The sideEffects property in package.json can help manage these situations, indicating that modules may have side effects that affect initialization.
In conclusion, understanding and managing recursive dependencies is a vital skill for frontend developers. By adopting best practices, utilizing tools, and reorganizing code structures, developers can navigate the maze of dependencies with confidence. The goal is to create a stable, maintainable codebase that stands the test of time. As the landscape of frontend development evolves, staying ahead of these challenges will ensure success in building robust applications.