Testing in Laravel: The Backbone of Reliable Applications
October 12, 2024, 10:23 am
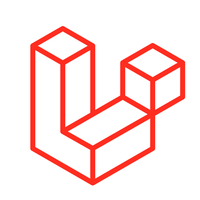
Location: United States, North Carolina, Cramerton
Employees: 51-200
Founded date: 2011
Total raised: $57M
In the world of web development, reliability is king. Developers need to ensure that their applications function smoothly, even when the unexpected occurs. Laravel, a popular PHP framework, provides a robust ecosystem for building web applications. But how do developers ensure that their code remains intact? The answer lies in testing. Testing in Laravel is not just a safety net; it’s a vital part of the development process. This article explores the different types of tests available in Laravel and their significance.
Laravel supports three primary types of tests: unit tests, integration tests, and functional tests. Each type serves a unique purpose, akin to different tools in a toolbox. Let’s break them down.
**Unit Tests: The Building Blocks**
Unit tests are the smallest tests in the Laravel ecosystem. They focus on individual components of the code, such as methods or classes. Think of them as the foundation of a house. If the foundation is weak, the entire structure is at risk.
Unit tests isolate specific pieces of code, ensuring that they perform as expected. For instance, if you have a method that calculates discounts, a unit test would check if it returns the correct value when given a specific input. This isolation is crucial. It allows developers to pinpoint issues quickly without the noise of external dependencies like databases or APIs.
A simple example of a unit test in Laravel might look like this:
```php
namespace Tests\Unit;
use PHPUnit\Framework\TestCase;
use App\Services\DiscountService;
class DiscountServiceTest extends TestCase {
public function test_calculate_discount() {
$price = 100;
$expectedDiscount = 90; // 10% discount
$service = new DiscountService();
$actualDiscount = $service->calculateDiscount($price);
$this->assertEquals($expectedDiscount, $actualDiscount);
}
}
```
This test checks if the discount calculation is accurate. If it fails, developers know exactly where to look.
**Integration Tests: The Connective Tissue**
Integration tests take a broader view. They examine how different components of the application work together. Imagine a team of musicians playing in harmony. Each musician (component) must perform well individually, but they also need to synchronize with one another.
In Laravel, integration tests might involve checking how a service interacts with a database or an external API. For example, if a service fetches data from an API and saves it to a database, an integration test would ensure that this entire process works seamlessly.
Here’s a glimpse of what an integration test might look like:
```php
namespace Tests\Feature;
use Tests\TestCase;
use Illuminate\Foundation\Testing\RefreshDatabase;
use App\Services\ExternalApiService;
class ApiIntegrationTest extends TestCase {
use RefreshDatabase;
public function test_external_api_data_is_saved_to_database() {
$this->mock(ExternalApiService::class, function ($mock) {
$mock->shouldReceive('fetchData')
->once()
->andReturn(['name' => 'Test Item', 'price' => 100]);
});
$response = $this->get('/api/fetch-data');
$response->assertStatus(200);
$this->assertDatabaseHas('items', ['name' => 'Test Item', 'price' => 100]);
}
}
```
This test checks if the data fetched from the API is correctly saved in the database. It’s a crucial step in ensuring that the application behaves as expected when components interact.
**Functional Tests: The User’s Perspective**
Functional tests are the final piece of the puzzle. They simulate user interactions with the application. Think of them as a dress rehearsal before the big show. They ensure that everything works together from the user’s perspective.
In Laravel, functional tests can be executed using tools like Laravel Dusk, which allows for browser testing. For instance, a functional test might check if a user can successfully log in to the application. Here’s how that might look:
```php
namespace Tests\Browser;
use Laravel\Dusk\Browser;
use Tests\DuskTestCase;
class LoginTest extends DuskTestCase {
public function test_user_can_login() {
$this->browse(function (Browser $browser) {
$browser->visit('/login')
->type('email', 'test@example.com')
->type('password', 'password')
->press('Login')
->assertPathIs('/home');
});
}
}
```
This test mimics a real user logging in. If it fails, developers know there’s a problem with the user experience.
**API Testing: The Digital Communication**
In today’s world, APIs are the lifeblood of applications. They allow different systems to communicate. Laravel makes it easy to test APIs, ensuring that they return the expected data. For example, a test might check if an API returns a list of products with the correct structure:
```php
namespace Tests\Feature;
use Tests\TestCase;
class ProductsApiTest extends TestCase {
public function test_get_products() {
$response = $this->getJson('/api/products');
$response->assertStatus(200)
->assertJsonStructure(['*' => ['id', 'name', 'price']]);
}
}
```
This test ensures that the API responds correctly, which is vital for maintaining a seamless user experience.
**Conclusion: The Safety Net**
Testing in Laravel is not just a luxury; it’s a necessity. Each type of test plays a crucial role in ensuring that applications run smoothly. Unit tests provide a solid foundation, integration tests ensure components work together, and functional tests simulate real user interactions. Together, they create a safety net that catches issues before they reach users.
In a world where reliability is paramount, embracing testing in Laravel is like building a fortress around your application. It protects against the unexpected and ensures that users have a seamless experience. So, whether you’re a seasoned developer or just starting, remember: testing is your best friend.
Laravel supports three primary types of tests: unit tests, integration tests, and functional tests. Each type serves a unique purpose, akin to different tools in a toolbox. Let’s break them down.
**Unit Tests: The Building Blocks**
Unit tests are the smallest tests in the Laravel ecosystem. They focus on individual components of the code, such as methods or classes. Think of them as the foundation of a house. If the foundation is weak, the entire structure is at risk.
Unit tests isolate specific pieces of code, ensuring that they perform as expected. For instance, if you have a method that calculates discounts, a unit test would check if it returns the correct value when given a specific input. This isolation is crucial. It allows developers to pinpoint issues quickly without the noise of external dependencies like databases or APIs.
A simple example of a unit test in Laravel might look like this:
```php
namespace Tests\Unit;
use PHPUnit\Framework\TestCase;
use App\Services\DiscountService;
class DiscountServiceTest extends TestCase {
public function test_calculate_discount() {
$price = 100;
$expectedDiscount = 90; // 10% discount
$service = new DiscountService();
$actualDiscount = $service->calculateDiscount($price);
$this->assertEquals($expectedDiscount, $actualDiscount);
}
}
```
This test checks if the discount calculation is accurate. If it fails, developers know exactly where to look.
**Integration Tests: The Connective Tissue**
Integration tests take a broader view. They examine how different components of the application work together. Imagine a team of musicians playing in harmony. Each musician (component) must perform well individually, but they also need to synchronize with one another.
In Laravel, integration tests might involve checking how a service interacts with a database or an external API. For example, if a service fetches data from an API and saves it to a database, an integration test would ensure that this entire process works seamlessly.
Here’s a glimpse of what an integration test might look like:
```php
namespace Tests\Feature;
use Tests\TestCase;
use Illuminate\Foundation\Testing\RefreshDatabase;
use App\Services\ExternalApiService;
class ApiIntegrationTest extends TestCase {
use RefreshDatabase;
public function test_external_api_data_is_saved_to_database() {
$this->mock(ExternalApiService::class, function ($mock) {
$mock->shouldReceive('fetchData')
->once()
->andReturn(['name' => 'Test Item', 'price' => 100]);
});
$response = $this->get('/api/fetch-data');
$response->assertStatus(200);
$this->assertDatabaseHas('items', ['name' => 'Test Item', 'price' => 100]);
}
}
```
This test checks if the data fetched from the API is correctly saved in the database. It’s a crucial step in ensuring that the application behaves as expected when components interact.
**Functional Tests: The User’s Perspective**
Functional tests are the final piece of the puzzle. They simulate user interactions with the application. Think of them as a dress rehearsal before the big show. They ensure that everything works together from the user’s perspective.
In Laravel, functional tests can be executed using tools like Laravel Dusk, which allows for browser testing. For instance, a functional test might check if a user can successfully log in to the application. Here’s how that might look:
```php
namespace Tests\Browser;
use Laravel\Dusk\Browser;
use Tests\DuskTestCase;
class LoginTest extends DuskTestCase {
public function test_user_can_login() {
$this->browse(function (Browser $browser) {
$browser->visit('/login')
->type('email', 'test@example.com')
->type('password', 'password')
->press('Login')
->assertPathIs('/home');
});
}
}
```
This test mimics a real user logging in. If it fails, developers know there’s a problem with the user experience.
**API Testing: The Digital Communication**
In today’s world, APIs are the lifeblood of applications. They allow different systems to communicate. Laravel makes it easy to test APIs, ensuring that they return the expected data. For example, a test might check if an API returns a list of products with the correct structure:
```php
namespace Tests\Feature;
use Tests\TestCase;
class ProductsApiTest extends TestCase {
public function test_get_products() {
$response = $this->getJson('/api/products');
$response->assertStatus(200)
->assertJsonStructure(['*' => ['id', 'name', 'price']]);
}
}
```
This test ensures that the API responds correctly, which is vital for maintaining a seamless user experience.
**Conclusion: The Safety Net**
Testing in Laravel is not just a luxury; it’s a necessity. Each type of test plays a crucial role in ensuring that applications run smoothly. Unit tests provide a solid foundation, integration tests ensure components work together, and functional tests simulate real user interactions. Together, they create a safety net that catches issues before they reach users.
In a world where reliability is paramount, embracing testing in Laravel is like building a fortress around your application. It protects against the unexpected and ensures that users have a seamless experience. So, whether you’re a seasoned developer or just starting, remember: testing is your best friend.