Bridging the Gap: Compiling C in JavaScript with Bun
September 29, 2024, 3:54 pm
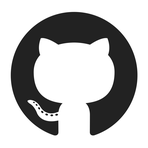
Location: United States, California, San Francisco
Employees: 1001-5000
Founded date: 2008
Total raised: $350M
In the world of programming, C is the backbone. It powers everything from file compression to network interactions. Even the browser you use to read this article relies on C. But what if you could harness the power of C directly from JavaScript? Enter Bun, a new player in the JavaScript ecosystem that allows developers to compile and execute native C code seamlessly.
Bun v1.1.28 introduces an experimental feature that lets you compile and run C code directly from JavaScript. This is a game-changer. Traditionally, developers faced two main routes to use system libraries in JavaScript: writing native add-ons using N-API or compiling code to WebAssembly (WASM). Both methods come with their own set of challenges.
N-API, while powerful, can be cumbersome. It requires a complex setup involving Python and C++ compilers, which can complicate continuous integration (CI) pipelines. The overhead of managing these dependencies can be a nightmare for developers. Each time a new version of Node.js is released, the risk of breaking changes looms large. The result? Increased complexity and frustration.
On the other hand, WebAssembly offers a way to run C code in a sandboxed environment. However, it comes with limitations. WebAssembly’s isolated memory model means that it cannot directly access system APIs. This leads to performance issues, as data must be cloned between JavaScript and WebAssembly, negating many of the performance benefits that WebAssembly promises.
Bun’s approach is different. It allows for direct compilation and execution of C code, eliminating the need for these convoluted workarounds. Imagine writing a simple C function, compiling it, and calling it from JavaScript with minimal overhead. That’s the promise of Bun.
Let’s look at a practical example. Suppose you want to create a random number generator in C and call it from JavaScript. With Bun, you can write a simple C function:
```c
#include
#include
int myRandom() {
return rand() + 42;
}
```
Now, in your JavaScript file, you can compile and execute this function with ease:
```javascript
import { cc } from "bun:ffi";
export const { symbols: { myRandom } } = cc({
source: "./myRandom.c",
symbols: {
myRandom: {
returns: "int",
args: [],
},
},
});
console.log(myRandom());
```
This simplicity is revolutionary. Bun uses TinyCC to compile the C code in memory, generating wrappers that convert JavaScript types to C types and vice versa. This means that you can call C functions as if they were native JavaScript functions, with minimal performance overhead.
Performance is a critical factor in programming. With Bun, the overhead of calling C functions is impressively low. Benchmarks show that calling a simple C function takes around 6 nanoseconds, compared to 4 nanoseconds for a native C call. This means that Bun’s FFI (Foreign Function Interface) adds only a slight overhead, making it a viable option for performance-critical applications.
But what can you build with this capability? The possibilities are vast. For instance, you can leverage existing C libraries for tasks like image processing, data compression, or even video encoding. Bun allows you to integrate these powerful libraries directly into your JavaScript applications without the usual hassle.
Consider video processing. Using libraries like FFmpeg, you can convert video formats directly from JavaScript. With Bun, you can compile the necessary C code and call it from your JavaScript application, achieving significant speed improvements. This could be a game-changer for web applications that require real-time video processing.
Moreover, Bun’s ability to handle dynamic linking with shared libraries opens up even more opportunities. You can create complex applications that rely on multiple C libraries, all while maintaining the simplicity of JavaScript. This means that developers can write high-performance applications without needing to dive deep into the intricacies of C programming.
However, it’s essential to note that while Bun simplifies the process, it doesn’t eliminate the need for a solid understanding of both C and JavaScript. Developers must still be mindful of memory management and type safety when working across these languages. But for those willing to embrace this new paradigm, the rewards are substantial.
In conclusion, Bun’s ability to compile and execute C code from JavaScript represents a significant leap forward in web development. It bridges the gap between high-level scripting and low-level programming, allowing developers to harness the full power of C without the usual complexities. As the tech landscape continues to evolve, tools like Bun will play a crucial role in shaping the future of programming, making it easier for developers to create powerful, efficient applications. The world of programming is changing, and Bun is at the forefront of this revolution.
Bun v1.1.28 introduces an experimental feature that lets you compile and run C code directly from JavaScript. This is a game-changer. Traditionally, developers faced two main routes to use system libraries in JavaScript: writing native add-ons using N-API or compiling code to WebAssembly (WASM). Both methods come with their own set of challenges.
N-API, while powerful, can be cumbersome. It requires a complex setup involving Python and C++ compilers, which can complicate continuous integration (CI) pipelines. The overhead of managing these dependencies can be a nightmare for developers. Each time a new version of Node.js is released, the risk of breaking changes looms large. The result? Increased complexity and frustration.
On the other hand, WebAssembly offers a way to run C code in a sandboxed environment. However, it comes with limitations. WebAssembly’s isolated memory model means that it cannot directly access system APIs. This leads to performance issues, as data must be cloned between JavaScript and WebAssembly, negating many of the performance benefits that WebAssembly promises.
Bun’s approach is different. It allows for direct compilation and execution of C code, eliminating the need for these convoluted workarounds. Imagine writing a simple C function, compiling it, and calling it from JavaScript with minimal overhead. That’s the promise of Bun.
Let’s look at a practical example. Suppose you want to create a random number generator in C and call it from JavaScript. With Bun, you can write a simple C function:
```c
#include
#include
int myRandom() {
return rand() + 42;
}
```
Now, in your JavaScript file, you can compile and execute this function with ease:
```javascript
import { cc } from "bun:ffi";
export const { symbols: { myRandom } } = cc({
source: "./myRandom.c",
symbols: {
myRandom: {
returns: "int",
args: [],
},
},
});
console.log(myRandom());
```
This simplicity is revolutionary. Bun uses TinyCC to compile the C code in memory, generating wrappers that convert JavaScript types to C types and vice versa. This means that you can call C functions as if they were native JavaScript functions, with minimal performance overhead.
Performance is a critical factor in programming. With Bun, the overhead of calling C functions is impressively low. Benchmarks show that calling a simple C function takes around 6 nanoseconds, compared to 4 nanoseconds for a native C call. This means that Bun’s FFI (Foreign Function Interface) adds only a slight overhead, making it a viable option for performance-critical applications.
But what can you build with this capability? The possibilities are vast. For instance, you can leverage existing C libraries for tasks like image processing, data compression, or even video encoding. Bun allows you to integrate these powerful libraries directly into your JavaScript applications without the usual hassle.
Consider video processing. Using libraries like FFmpeg, you can convert video formats directly from JavaScript. With Bun, you can compile the necessary C code and call it from your JavaScript application, achieving significant speed improvements. This could be a game-changer for web applications that require real-time video processing.
Moreover, Bun’s ability to handle dynamic linking with shared libraries opens up even more opportunities. You can create complex applications that rely on multiple C libraries, all while maintaining the simplicity of JavaScript. This means that developers can write high-performance applications without needing to dive deep into the intricacies of C programming.
However, it’s essential to note that while Bun simplifies the process, it doesn’t eliminate the need for a solid understanding of both C and JavaScript. Developers must still be mindful of memory management and type safety when working across these languages. But for those willing to embrace this new paradigm, the rewards are substantial.
In conclusion, Bun’s ability to compile and execute C code from JavaScript represents a significant leap forward in web development. It bridges the gap between high-level scripting and low-level programming, allowing developers to harness the full power of C without the usual complexities. As the tech landscape continues to evolve, tools like Bun will play a crucial role in shaping the future of programming, making it easier for developers to create powerful, efficient applications. The world of programming is changing, and Bun is at the forefront of this revolution.