Building Applications with NestJS and Angular: A Guide to Deployment with PM2 and Docker Compose
September 1, 2024, 3:49 pm
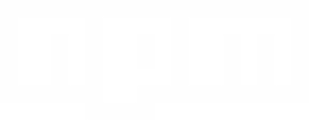
Location: United States, California, Oakland
Employees: 11-50
Founded date: 2009
Total raised: $8M
In the world of web development, building applications is like crafting a fine piece of art. Each layer, each component, must fit together seamlessly. This article dives into the intricacies of deploying applications built with NestJS and Angular, focusing on two popular methods: PM2 and Docker Compose.
NestJS is a powerful framework for building server-side applications, while Angular shines on the client side. Together, they create a robust full-stack solution. But how do you get these applications from your local environment to production? Let’s explore.
### Setting the Stage
Before diving into deployment, ensure you have the necessary tools. You’ll need Node.js, npm, and Docker installed. Think of these as your paintbrushes and palette. Without them, you can’t create your masterpiece.
### Step 1: Preparing Your Application
First, you need to prepare your application for deployment. This involves installing dependencies and generating necessary files. Run the following command to install the required packages:
```bash
npm install --save @nestjs/serve-static dotenv wait-on
```
This command sets the stage, ensuring your application has the tools it needs to serve static files and manage environment variables.
### Step 2: Configuring NestJS for Static Files
NestJS can serve static files, making it easy to integrate your Angular frontend. You’ll need to modify your `app.module.ts` file to include the `ServeStaticModule`. This module allows NestJS to serve your Angular build files directly.
Here’s a snippet to guide you:
```typescript
import { ServeStaticModule } from '@nestjs/serve-static';
import { join } from 'path';
@Module({
imports: [
ServeStaticModule.forRoot([
{
rootPath: join(__dirname, '..', 'client'), // Adjust the path as necessary
},
]),
],
})
export class AppModule {}
```
This code tells NestJS where to find your static files. It’s like giving directions to a delivery driver.
### Step 3: Setting Up PM2 for Process Management
PM2 is a process manager for Node.js applications. It’s like a conductor leading an orchestra, ensuring everything runs smoothly. To set up PM2, create a configuration file named `ecosystem.config.js`:
```json
{
"apps": [
{
"name": "nestjs-angular-app",
"script": "dist/main.js",
"watch": true,
"env": {
"NODE_ENV": "production"
}
}
]
}
```
This file tells PM2 how to run your application. The `watch` option allows PM2 to restart your app automatically when changes are detected.
### Step 4: Building Your Angular Application
Next, you need to build your Angular application. This step compiles your Angular code into static files that can be served by NestJS. Run:
```bash
ng build --prod
```
This command creates a `dist` folder containing your compiled Angular files. Think of it as the final brush strokes on your canvas.
### Step 5: Dockerizing Your Application
Docker allows you to package your application and its dependencies into a container. This ensures your application runs consistently across different environments. Create a `Dockerfile` in your project root:
```dockerfile
FROM node:14
WORKDIR /usr/src/app
COPY package*.json ./
RUN npm install
COPY . .
EXPOSE 3000
CMD ["npm", "run", "start:prod"]
```
This Dockerfile sets up a Node.js environment, installs dependencies, and specifies how to run your application.
### Step 6: Creating a Docker Compose File
Docker Compose simplifies the process of managing multi-container applications. Create a `docker-compose.yml` file:
```yaml
version: '3'
services:
app:
build: .
ports:
- "3000:3000"
environment:
NODE_ENV: production
```
This file defines your application service, mapping port 3000 on your host to port 3000 in the container. It’s like opening a window to let fresh air into your home.
### Step 7: Running Your Application
Now, you’re ready to run your application. If you’re using PM2, execute:
```bash
pm2 start ecosystem.config.js
```
For Docker, run:
```bash
docker-compose up --build
```
Both commands will start your application, making it accessible to users.
### Step 8: Testing Your Application
Once your application is running, it’s crucial to test it. Ensure all routes work as expected. Use tools like Postman or your browser to interact with your API and frontend. This step is like stepping back to admire your artwork, ensuring every detail is perfect.
### Conclusion
Deploying applications with NestJS and Angular using PM2 and Docker Compose is a powerful approach. Each step is essential, from preparing your application to testing it in production. With these tools, you can ensure your applications are robust, scalable, and ready for users.
Remember, deployment is not the end. It’s just the beginning of your application’s journey. Keep monitoring, updating, and improving. Your masterpiece deserves it.
NestJS is a powerful framework for building server-side applications, while Angular shines on the client side. Together, they create a robust full-stack solution. But how do you get these applications from your local environment to production? Let’s explore.
### Setting the Stage
Before diving into deployment, ensure you have the necessary tools. You’ll need Node.js, npm, and Docker installed. Think of these as your paintbrushes and palette. Without them, you can’t create your masterpiece.
### Step 1: Preparing Your Application
First, you need to prepare your application for deployment. This involves installing dependencies and generating necessary files. Run the following command to install the required packages:
```bash
npm install --save @nestjs/serve-static dotenv wait-on
```
This command sets the stage, ensuring your application has the tools it needs to serve static files and manage environment variables.
### Step 2: Configuring NestJS for Static Files
NestJS can serve static files, making it easy to integrate your Angular frontend. You’ll need to modify your `app.module.ts` file to include the `ServeStaticModule`. This module allows NestJS to serve your Angular build files directly.
Here’s a snippet to guide you:
```typescript
import { ServeStaticModule } from '@nestjs/serve-static';
import { join } from 'path';
@Module({
imports: [
ServeStaticModule.forRoot([
{
rootPath: join(__dirname, '..', 'client'), // Adjust the path as necessary
},
]),
],
})
export class AppModule {}
```
This code tells NestJS where to find your static files. It’s like giving directions to a delivery driver.
### Step 3: Setting Up PM2 for Process Management
PM2 is a process manager for Node.js applications. It’s like a conductor leading an orchestra, ensuring everything runs smoothly. To set up PM2, create a configuration file named `ecosystem.config.js`:
```json
{
"apps": [
{
"name": "nestjs-angular-app",
"script": "dist/main.js",
"watch": true,
"env": {
"NODE_ENV": "production"
}
}
]
}
```
This file tells PM2 how to run your application. The `watch` option allows PM2 to restart your app automatically when changes are detected.
### Step 4: Building Your Angular Application
Next, you need to build your Angular application. This step compiles your Angular code into static files that can be served by NestJS. Run:
```bash
ng build --prod
```
This command creates a `dist` folder containing your compiled Angular files. Think of it as the final brush strokes on your canvas.
### Step 5: Dockerizing Your Application
Docker allows you to package your application and its dependencies into a container. This ensures your application runs consistently across different environments. Create a `Dockerfile` in your project root:
```dockerfile
FROM node:14
WORKDIR /usr/src/app
COPY package*.json ./
RUN npm install
COPY . .
EXPOSE 3000
CMD ["npm", "run", "start:prod"]
```
This Dockerfile sets up a Node.js environment, installs dependencies, and specifies how to run your application.
### Step 6: Creating a Docker Compose File
Docker Compose simplifies the process of managing multi-container applications. Create a `docker-compose.yml` file:
```yaml
version: '3'
services:
app:
build: .
ports:
- "3000:3000"
environment:
NODE_ENV: production
```
This file defines your application service, mapping port 3000 on your host to port 3000 in the container. It’s like opening a window to let fresh air into your home.
### Step 7: Running Your Application
Now, you’re ready to run your application. If you’re using PM2, execute:
```bash
pm2 start ecosystem.config.js
```
For Docker, run:
```bash
docker-compose up --build
```
Both commands will start your application, making it accessible to users.
### Step 8: Testing Your Application
Once your application is running, it’s crucial to test it. Ensure all routes work as expected. Use tools like Postman or your browser to interact with your API and frontend. This step is like stepping back to admire your artwork, ensuring every detail is perfect.
### Conclusion
Deploying applications with NestJS and Angular using PM2 and Docker Compose is a powerful approach. Each step is essential, from preparing your application to testing it in production. With these tools, you can ensure your applications are robust, scalable, and ready for users.
Remember, deployment is not the end. It’s just the beginning of your application’s journey. Keep monitoring, updating, and improving. Your masterpiece deserves it.